How to Develop a Microservices-Based Architecture with Python – The Deep Dive
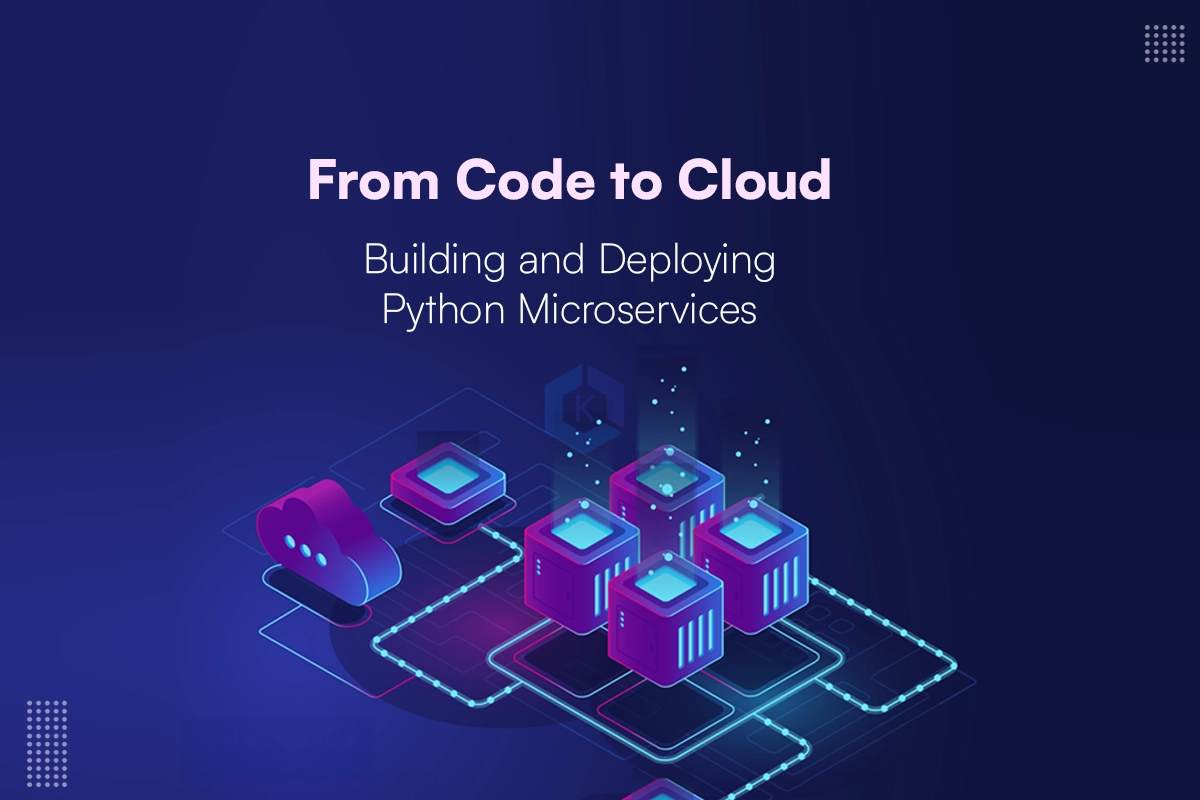
In the Previous Section of our microservices journey, we explored the shift from monolithic architectures, the foundational principles of microservices, and how to set up and build individual services using Python. With that groundwork laid, it’s time to level up – let’s diving into service-to-service communication, efficient data management, and real-world deployment strategies using AWS. These next steps will help transform your microservices into a scalable, production-ready system.
Building a Simple Microservice with Python
As you see our project is already set up, now let’s build a basic microservice.
Setting up a REST API using FastAPI or Django REST Framework (DRF)
The first step is to expose an API that allows external services to interact with our microservice. The two most efficient ways to do this in Python are: FastApi and DRF
- FastAPI –It’s Ideal for performance and async operations. And it automatically generates OpenAPI documentation!
FastAPI Example :
Run the API:
- Django REST Framework (DRF) – Its always great choice if you want structured framework and need built-in authentication, ORM or if your microservice needs database models, authentication, or admin support.
Install DRF:
Add to settings.py
Create a Simple API in Django
Defining API endpoints and data models
Microservices handle structured data, so we need to define data models. Here’s an example User API:
FastAPI (Using Pydantic for Validation)
Django (Using Django ORM)
Django ORM handles database interactions easily.
Implementing authentication
Securing microservices is essential, and authentication plays a key role .OAuth2 and JWT (JSON Web Tokens) are among the most widely used methods to protect endpoints effectively.
FastAPI Authentication with JWT
OAuth2PasswordBearer handles JWT token authentication.
Django Authentication with DRF
Using PyTest for API testing
Automated testing ensures microservices work correctly. PyTest makes API testing easy.
Install PyTest & Requests
Basic PyTest Example for FastAPI
Service-to-Service Communication and Data Management
In a microservices architecture, seamless communication between services and efficient data management are essential for maintaining performance, scalability, and consistency.Let’s explore how services talk to each other and manage data without stepping on each other’s toes.
REST APIs vs. Asynchronous Messaging (Kafka, RabbitMQ).
Microservices can communicate using two primary methods:
- REST APIs (Synchronous Communication): As we seen, Use REST APIs for quick and real-time responses. For more flexible,non-blocking communication, message queues are a better fit .they decouple services and improve scalability.
- Asynchronous Messaging (Event-Driven Communication): Tools like Kafka and RabbitMQ let services talk without waiting for replies-events are published to a queue and processed later which reducing dependencies and boost scalability
Implementing gRPC for High-Performance Communication
gRPC offers faster, binary-based communication between microservices by using Protocol Buffers instead of JSON, making it much more efficient than REST.
Key benefits of gRPC:
Lightweight and efficient – Faster than traditional REST APIs.
Supports multiple programming languages – Allows cross-platform service communication.
Ideal for real-time, high-frequency interactions – Used in performance-intensive applications.
Database per service: PostgreSQL, MySQL, MongoDB.
Each microservice should use its own database to stay independent and scale easily.
- Relational Databases (PostgreSQL, MySQL) – Suits for structured data where relationships between tables are needed. PostgreSQL and MySQL are popular for transactional data and complex queries.
- NoSQL Databases (MongoDB, DynamoDB) – Best for unstructured or semi-structured data. These databases offer flexibility, scalability, and high availability and handling large-scale applications.
Real-World Python Tools: Celery for Background Tasks
Celery is a powerful Python tool for running tasks asynchronously – It’s perfect for things like sending emails, generating reports, or scheduled jobs. It helps microservices stay responsive by handling long-running tasks in the background. It’s especially useful when you need to scale and distribute work across multiple workers without blocking the main service.
Deploying Python Microservices on AWS
Once your microservices are ready, it’s time to get them live. AWS makes it super easy to deploy, scale, and manage everything with containers.
Setting Up AWS EKS for Containerized Microservices
Amazon Elastic Kubernetes Service (EKS) simplifies running Kubernetes clusters on AWS, making it a great choice for managing containerized microservices. With EKS, you get:
Automated Kubernetes management – No need to manually manage control planes.
Integration with AWS services – Works seamlessly with IAM, VPC, and CloudWatch.
Built-in scaling and high availability – Kubernetes-based microservices can dynamically scale.
Key Steps to Deploy on EKS:
- Containerize your microservices using Docker.
- Deploy them using Kubernetes manifests or Helm charts.
- Use Cloud Launchpad to streamline Kubernetes-based deployments on AWS.
Automating Deployments with CI/CD
Continuous Integration and Continuous Deployment (CI/CD) ensure microservices are deployed quickly and consistently. AWS provides several options for automation:
AWS CodePipeline + CodeBuild – Fully managed CI/CD for automating builds, tests, and deployments.
Jenkins, GitHub Actions, or GitLab CI – Popular tools for managing deployment pipelines.
ArgoCD or Flux – GitOps-based continuous deployment for Kubernetes.
Pro tip: Use Infrastructure as Code tools like Terraform or AWS CloudFormation to set up and manage your AWS stuff—it saves a ton of time and keeps things clean.
Auto-scaling and load balancing made easy
Your microservices should grow (or shrink) with traffic, right? AWS has your back with tools like:
- HPA – It adds more pods when things get busy.
- ALB – Think of it as the traffic cop, sending requests to the right service.
- Cluster Autoscaler – Spins up more nodes when your cluster needs them.
All this means your app stays fast, available, and smooth—even when things get wild.
Security and Observability
In any application, security is always a top concern,they’re built into the architecture. With each service isolated, it’s easier to control access, contain issues, and monitor performance in real-time. Compared to monolithic apps, microservices offer stronger fault isolation and better visibility across the system.
API Security Features in Microservices
Your microservices usually expose APIs -REST or gRPC -and those need to be protected. Here’s how to keep unwanted guests out:
- OAuth2 & JWT Authentication – Token-based authentication that’s secure and flexible.
- Role-Based Access Control – It Restricts access based on user roles, limiting exposure.
- Rate Limiting & Throttling – Controls excessive requests to prevent abuse.
- API Gateway Enforcement – Implements authentication, validation, and monitoring before traffic reaches services.
Use AWS API Gateway to handle auth, validation, and monitoring right at the door.
Secure Access Management with AWS
Managing sensitive credentials and access control is essential in microservices. AWS offers:
- AWS IAM – Defines access permissions for users and services.
- AWS Secrets Manager – Store API keys, DB credentials, and sensitive data securely.
- AWS KMS – Encrypts sensitive data using managed encryption keys.
Best Practice is Never hardcode credentials. Use AWS Secrets Manager to retrieve them dynamically.
Service-to-Service Security with Istio
In a microservice, services talk to each other alot. Istio, your service mesh buddy
- Mutual TLS (mTLS) – Encrypts data between microservices.
- Fine-Grained Access Control – Blocks communication between services.
- Traffic Monitoring– Helps you understand (and fix) what’s happening between services.
Best Practice is use Istio on your Kubernetes cluster for built-in security
Real-Time Monitoring with Prometheus and Grafana
Microservices generate large amounts of data,and you need good tools that help you spot issues, understand performance, and stay ahead of problems.
- Prometheus collects real-time metrics (CPU usage, memory, request latency).
- Grafana visualizes performance data with cool dashboards.
- Alerts kick in when things start to slow down or break.
Best Practice is Use Prometheus with Grafana to track microservices performance in real-time, Just like Cloud Launchpad do.
Centralized Logging with AWS CloudWatch
When you’ve got lots of microservices running, they each generate logs and things can get messy fast. Centralized logging brings all that data together,easy to debug and stay on top of issues.
- Log Aggregation – Pull logs from all services in one place.
- Log Filtering & Analysis – Helps find issues faster.
- Automated Alerts – Notifies teams of critical failures.
By implementing these CloudWatch keeps your system more secure, resilient, and easier to manage.
Conclusion:
Building a scalable, efficient, and secure microservices architecture with Python is all about making the right design choices and using the best tools.
In this article we’ve covered how to set up a Python-based microservices project, and key best practices for everything from deployment to security.
Key Takeaways for Scalable Microservices
- Use the right framework – FastAPI for high-performance APIs, Django for full-featured applications, and Flask for lightweight services.
- Ensure service independence – Decouple services to improve scalability and fault isolation.
- Adopt containerization – Docker and Kubernetes simplify deployment and scaling.
- Secure APIs and infrastructure – Implement OAuth2, JWT, IAM policies, and API gateways.
- Enable observability – Use Prometheus, Grafana, and AWS CloudWatch for real-time monitoring.
Common Pitfalls to Avoid
- Tightly Coupled Services – Keep services independent to avoid one failure bringing everything down.
- Ignoring Observability – Without monitoring and logging, debugging in a distributed system becomes very difficult.
- Skipping API Versioning – Always version your APIs to avoid breaking things for users.
- Bad Database Strategy – Use a database-per-service model when necessary instead of a shared database.
- Security Gaps – Never ever hardcode secrets.always use proper encryption and access controls.
Meet CloudLaunchPad: Your Gateway to Effortless Infrastructure Automation for Scalable Microservices!
To ensure smooth deployments, automate CI/CD pipelines using tools like GitHub Actions, Jenkins, or GitLab CI/CD. Deploying microservices on AWS EKS provides scalability, and using CloudLaunchpad can further simplify the process.
Cloud Launchpad automates project deployment on EKS, handling cluster creation, load balancing, and instance management—reducing manual effort and making deployment seamless.
By following these best practices, adopting automation, and continuously monitoring and securing microservices, you can build a robust and future-ready architecture for scalable applications.