GraphQL vs REST: Choosing the Right API Development for Your Application
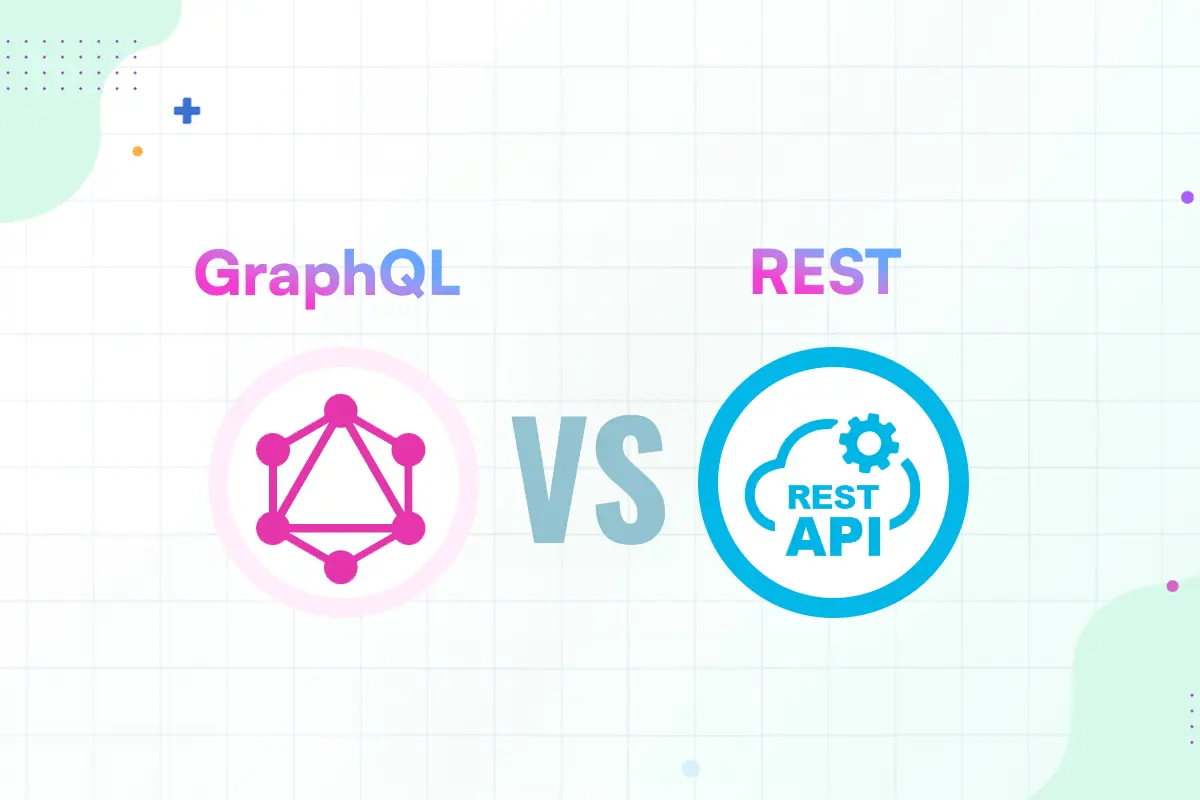
Introduction to GraphQL vs REST
In the world of web development, picking the right API design is crucial for how well your application performs and scales. Two popular options are REST (Representational State Transfer) and GraphQL, each with its own strengths that developers find useful.
GraphQL and REST are two distinct approaches to designing APIs for exchanging data over the internet. REST uses standard HTTP methods (GET, POST, PUT, DELETE) with dedicated endpoints for different resources, promoting a straightforward and stateless communication style.GraphQL, on the other hand, allows clients to request exactly the data they need through a single endpoint, making data fetching more efficient and flexible.
Both GraphQL and REST are essential technologies used in many modern applications. By understanding their individual strengths and weaknesses, you can better decide which API solution is best for your project.
What is GraphQL?
GraphQL is a query language for APIs and a runtime for fulfilling those queries with your existing data. GraphQL provides a complete and understandable description of the data in your API, gives clients the power to ask for exactly what they need and nothing more, makes it easier to evolve APIs over time, and enables powerful developer tools.
Key Concepts of GraphQL
a. Schema
At the core of any GraphQL API lies its schema. The schema defines the types of data that can be queried and serves as a contract between the client and the server, ensuring that clients can only request data that the server is capable of providing. This schema-driven approach ensures consistency and clarity in the data exchange process.
b. Queries
GraphQL queries empower clients to define the exact data they require, thus eliminating the common REST API issues of over-fetching and under-fetching. This precise data retrieval ensures efficiency and optimizes network performance.
c. Mutations
While queries are focused on retrieving data, mutations are designed to modify it. They operate similarly to queries but are intended for creating, updating, or deleting resources, enabling dynamic interaction with the data.
d. Resolvers
Resolvers are specialized functions responsible for retrieving the data requested in each field of a query. They can pull data from a variety of sources, including databases, other APIs, or any external data store, ensuring the requested information is accurately provided to the client.
Advantages of GraphQL
a. Efficient Data Fetching
GraphQL allows clients to request exactly the data they need, avoiding over-fetching and under-fetching issues common with REST. This results in more efficient use of network resources and faster application performance.
b. Single Endpoint
With GraphQL, all data requests are made to a single endpoint. This simplifies API design and reduces the complexity of managing multiple endpoints, making it easier to evolve and maintain the API.
c. Flexibility
GraphQL provides a high degree of flexibility by allowing clients to specify precisely the data they require. This adaptability is particularly beneficial in applications where data needs can vary significantly between different components or screens.
d. Strong Typing
GraphQL schemas are strongly typed, meaning that the structure and types of the data are explicitly defined. This ensures consistency and reliability in the API, enabling robust validation and error handling.
Disadvantages of GraphQL
a. Complexity
GraphQL introduces additional complexity, particularly in the initial setup and schema design. Developers need to learn and implement the GraphQL specification, which can be more challenging than working with traditional REST APIs.
b. Caching Challenges
Caching can be more complex with GraphQL compared to REST. In REST, caching is typically straightforward because each endpoint represents a specific resource. With GraphQL’s single endpoint, implementing efficient caching strategies requires more effort and careful planning.
c. Overhead
GraphQL queries can result in higher server overhead compared to REST, especially if clients request large amounts of data or complex nested queries. This can lead to increased processing time and resource consumption on the server.
Choosing the Right One
1.Project Requirements
If your application needs real-time data updates (e.g., a live chat application) or involves complex systems where clients need to request specific data sets, GraphQL might be the better choice. For simpler applications where standard CRUD (Create, Read, Update, Delete) operations are sufficient, REST could be more appropriate.
2. Factors to Consider
When deciding between REST and GraphQL, it’s important to evaluate your project’s specific requirements, such as the type of data, expected traffic, and your team’s familiarity with the technology.
3. Use Cases
REST is ideal for projects where data can be neatly categorized into resources and a well-defined structure is required. On the other hand, GraphQL shines in scenarios where flexibility in data retrieval is paramount and the data structures are complex or continually evolving.
4. Performance
Consider the performance implications of your choice. REST’s stateless nature and caching capabilities can boost performance in certain scenarios. Conversely, GraphQL’s ability to eliminate over-fetching can improve response times by ensuring clients receive only the data they need.
5. Team Expertise
Assess your team’s expertise. If your developers are already proficient in REST or GraphQL, it may be advantageous to stick with what they know to save time and resources. Training a team on a new technology can be time-consuming and may introduce initial inefficiencies.
What is REST?
REST, or Representational State Transfer, is an architectural style designed to provide standards for web communication, facilitating easier interaction between computer systems. It operates on a stateless, client-server model, utilizing the HTTP protocol to perform CRUD (Create, Read, Update, Delete) operations on data.
Key Characteristics of REST:
- Client-server architecture: In REST, there’s a clear division between how users interact with the interface and where data is stored. This separation improves the ability to use the same interface across different devices and platforms.
- Stateless: REST APIs are stateless, meaning servers do not retain client-specific information between requests, ensuring each request is handled independently.
- Cacheability: Responses in REST APIs can be designated as cacheable or non-cacheable, optimizing performance by allowing clients to reuse stored responses.
- Uniform interface: A uniform interface provides a standardized method to interact with the server, ensuring consistent access to resources across different devices and applications using HTTP methods.
How REST works
REST APIs use unique resource identifiers (URIs) to address resources and perform CRUD (Create, Read, Update, Delete) operations through different endpoints. These APIs rely on predefined data formats, known as media types or MIME types, to determine the structure and size of the resources provided to clients. Common formats include JSON and XML, though HTML and plain text are also used.
For example:
- A GET request retrieves a record.
- A POST request creates a new record.
- A PUT request updates an existing record.
- A DELETE request removes a record.
All HTTP methods can be utilized in API calls, making a well-designed REST API similar to a website running in a web browser with built-in HTTP functionality.
The state of a resource at any given moment is known as the resource representation. This representation can be delivered to a client in various formats, including JSON, HTML, XML, Python, PHP, or plain text. JSON is particularly popular due to its readability by both humans and machines and its programming language-agnostic nature.
Request headers and parameters are crucial in REST API calls as they include essential identifier information such as metadata, authorizations, URIs, caching, and cookies. Request and response headers, along with conventional HTTP status codes, are integral to well-designed REST APIs.
When a client requests a resource, the server processes the request and returns the associated data. The response typically includes HTTP status codes, such as:
- 200 OK: The request was successful, and the server is returning the requested resource.
- 404 Not Found: The requested resource does not exist on the server.
By adhering to these principles and utilizing standard HTTP methods and status codes, REST APIs provide a robust and flexible way to interact with networked resources.
Common use cases
- Web Services and APIs: RESTful APIs are widely used to expose web service functionalities. For instance, social media platforms like Twitter and Facebook offer REST APIs for third-party integration.
- Microservices Architecture: Microservices often communicate with each other using REST APIs. Each microservice exposes a RESTful interface, enabling independent development, deployment, and scaling.
- Mobile Applications: Mobile apps frequently use RESTful APIs to communicate with backend servers, such as a weather app fetching data from a remote server.
- Cloud Services: Cloud providers like AWS, Google Cloud, and Azure offer RESTful APIs for managing resources, such as creating virtual machines or deploying applications.
- E-commerce Applications: E-commerce platforms use REST APIs to manage operations like product listings, user accounts, shopping carts, and order processing.
Advantages of REST
REST is preferable to SOAP for several reasons. Here are a few advantages that REST APIs have:
- Simplicity:
REST APIs are designed to be simple and easy to use. They leverage standard HTTP methods, such as GET, POST, PUT, and DELETE, which are familiar to most developers. The use of URIs to access resources makes the API intuitive and easy to understand, reducing the learning curve and facilitating quick implementation.
- Scalability:
REST’s separation of client and server allows for easy scalability. Development teams can scale the application without significant challenges.
- Flexibility and Portability:
REST APIs facilitate data transfers between servers. They support database migrations and allow for changes in database structure without disrupting operations.
- Independence:
The client-server separation in REST promotes independent development across projects. Developers can work on different parts of the project concurrently, adapting APIs to various platforms and syntaxes.
- Lightweight:
REST APIs are lightweight and fast, leveraging HTTP standards that support multiple formats such as JSON, XML, and HTML. This efficiency makes them suitable for mobile apps, IoT devices, and diverse technological environments.
Disadvantages of REST
i. Over-fetching and Under-fetching
One of the main drawbacks of REST is the issue of over-fetching and under-fetching. Over-fetching occurs when a client retrieves more data than needed, while under-fetching happens when the client doesn’t get enough data in a single request, requiring multiple requests to gather all the necessary information. This can lead to inefficiencies and increased latency.
ii. Multiple Endpoints
In a RESTful system, different resources are accessed through distinct endpoints. As the number of resources grows, so does the number of endpoints. Managing and documenting a large number of endpoints can become cumbersome, leading to increased complexity and potential maintenance challenges.
iii. Rigid Structure
The structure of REST APIs can be somewhat rigid due to the reliance on fixed endpoints and HTTP methods. This rigidity can make it challenging to handle complex queries or interactions that do not fit neatly into the CRUD paradigm. Additionally, evolving the API over time to accommodate new features or changes can require significant effort, as changes might necessitate updates to multiple endpoints and clients
Wrap up
Both REST and GraphQL offer compelling features for API development, each with its own set of advantages and challenges. REST is renowned for its simplicity, scalability, and universality, making it suitable for many web applications. In contrast, GraphQL excels in precise data fetching, real-time updates, and efficiently handling complex systems, making it ideal for applications requiring flexibility and efficiency in data retrieval.
GraphQL facilitates rapid and efficient development by offering a declarative and adaptable environment, presenting significant advancements beyond the capabilities of REST. It has a large community, a vibrant ecosystem, and has been implemented in several popular languages, such as JavaScript, Go, and Java.
If you are aiming to develop an API for a mobile application, GraphQL should be your first option due to its efficient bandwidth usage. On the other hand, if your application requires a robust API with caching and a monitoring system, REST is the better choice.
The success of your project largely depends on how well your API handles data and queries. Whether you opt for REST or GraphQL, it’s essential to design your API thoughtfully and efficiently to meet your application’s specific needs. Staying informed about the latest trends and technologies in web development is key to making well-informed decisions that drive your project forward.