Setting Up Django as the Gateway
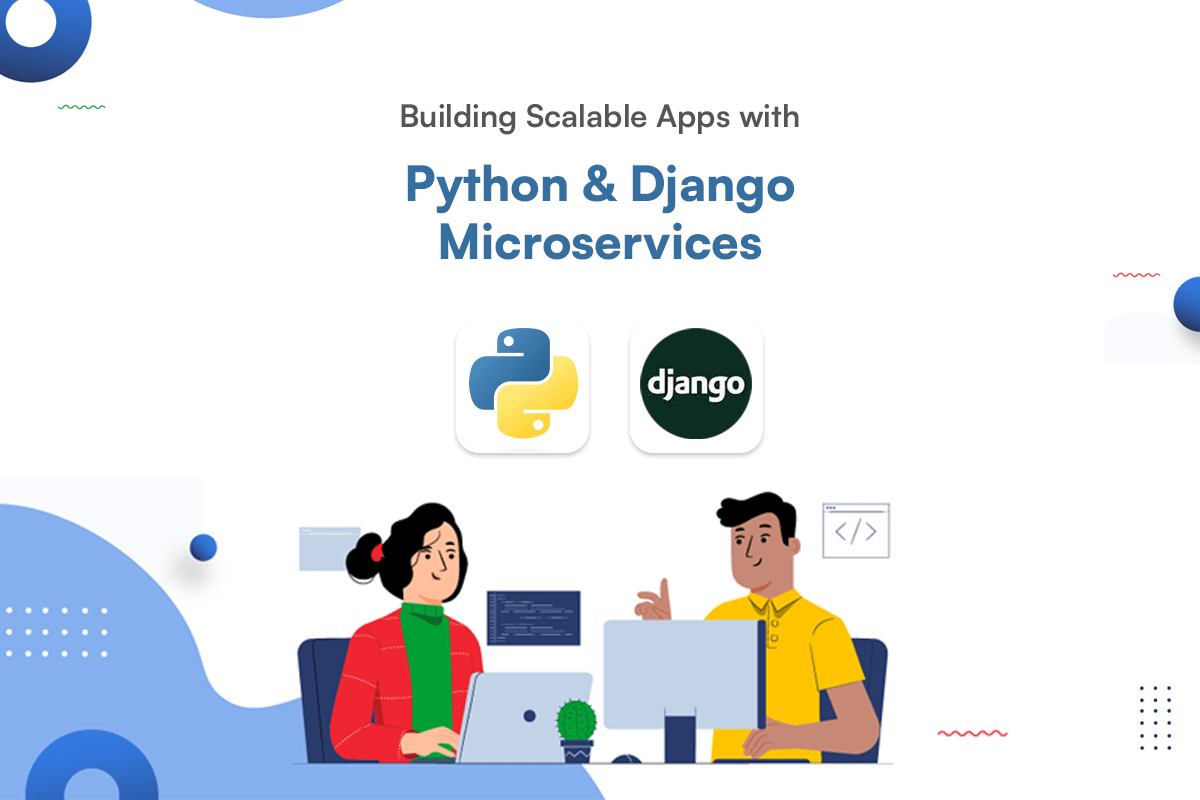
In our microservices architecture, Django will serve as the gateway responsible for routing requests to the appropriate microservices. Let’s go through the steps of setting up Django as the gateway.
Project Structure
Let’s begin by organizing our projects:
- Gateway Project:
- Project Name: gateway_project
- Django App: gateway_app
- User Microservice Project:
- Project Name: user_project
- Django App: users_app
- Product Microservice Project:
- Project Name: product_project
- Django App: products_app
Step 1: Create folder of MS
Step 2: Start Project and App for Gateway MS
Step 3: Start Project and App for User MS
Step 4: Start Project and App for Product MS
Step 5: Install Django Rest Framework
Step 6: Configure Django Settings For Gateway MS
Update the INSTALLED_APPS in settings.py to include ‘rest_framework’:
Step 7: Configure URLs for Gateway MS
gateway_project/urls.py
Step 8: Configure Business Logic for Gateway MS
gateway_project/gateway/views.py
Create a views.py file and define views that will communicate with the microservices:
Step 9: Configure Django Settings For User MS
user_project/settings.py
Step 10: Configure URLs for User MS
user_project/urls.py
Step 11: Configure Business Logic for User MS
user_project/users/views.py
Step 12: Configure Django Settings For Product MS
product_project/settings.py
Step 13: Configure URLs for Product MS
product_project/urls.py
Step 14: Configure Business Logic for User MS
product_project/products/views.py
Step 15 Configure Models.py file for User MS
user_project/users/models.py
Step 16 Configure Models.py file for Product MS
product_project/products/models.py
Running and Scaling Microservices
Step 1: Run Microservices Locally
- Start the User Microservice:
Parallelly start the Product microservice in different terminal:
Parallelly start the gateway microservice in different terminal:
Now, the gateway project should be accessible at http://localhost:8000/. It will route requests to the user microservice (http://localhost:8001/api/users/) and the product microservice (http://localhost:8002/api/products/). Adjust the URLs and ports as needed based on your setup.
Step 2: Scaling Microservices
To scale microservices, consider the following steps:
- Deploy microservices on separate servers or containers.
- Utilize container orchestration tools like Docker Compose or Kubernetes for managing multiple instances.
- Implement load balancing to distribute incoming traffic across multiple instances of a microservice.
Conclusion
In this comprehensive guide, we explored the foundations of microservices architecture and demonstrated how to implement a microservices system using Python Django as the gateway and Django Rest Framework for communication. The modular and independent nature of microservices provides flexibility, scalability, and resilience, making them an ideal choice for modern web applications.
As you delve deeper into microservices, you may encounter challenges such as service discovery, load balancing, and event-driven communication. Additionally, consider exploring advanced topics like API versioning, authentication, and authorization within a microservices context.
Microservices offer a powerful solution for building robust and scalable applications in today’s dynamic development landscape. By following the steps outlined in this blog, you’ll gain a solid understanding of microservices and be well-equipped to embark on your microservices journey.
Feel free to customize and expand upon this content based on your specific preferences and the depth you want to go into each section. Additionally, include code snippets, diagrams, and real-world examples to make the content more engaging and informative.
References
https://www.djangoproject.com/
https://www.w3schools.com/python/python_intro.asp
https://www.geeksforgeeks.org/python-programming-language/