Real Time Chat Application Using Django Channels
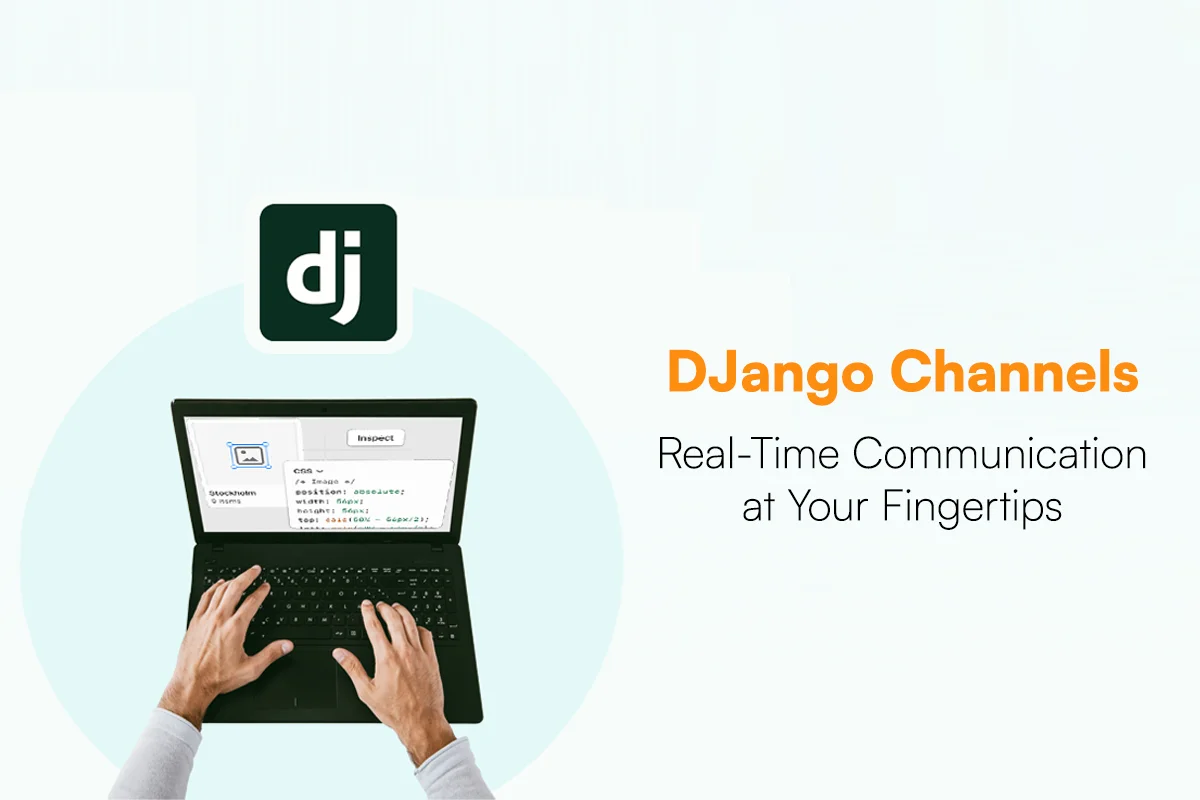
What are Django Channels?
Channels or Django channels is an extension which gives us flexibility to build applications which require real time communications, asynchronous tasks and events.
We often come across situations where there is a demand for a high end application which transfers data in real time. We can not rely on refreshing applications every-time in order to fetch new data.
Django channels let us build these applications which demand protocols beyond HTTP/HTTPS like websocket.
Components of django channels:
- ASGI interface: An interface which is responsible for handling long lived connections and asynchronous tasks.
- Consumers: They are similar to what we have in views but are for handling asynchronous tasks. They listen for events.
- Routing: Routing handles which URL will handle which consumer will handle a connection.
- Websocket handling: These are responsible for creating a connection, disconnecting a connection, receiving and sending messages.
When to use Django channels?
Django channels seem fancy and intriguing, but it must be understood that if any application that totally deals with synchronous requests, should not implement django channels.
If we are dealing with real time applications that are dealing with transfer of data in real time, django channels can come in handy.
Tasks which are asynchronous, meaning which can run in the background, require django channels.
Using django channels can unnecessarily complicate the structure of the whole application.
Use of channels
Real time chat application:-
How annoying it would be if for every message that we send, it needs a refresh in order to be displayed.
No one would use such a platform and let alone a whole organization for whom time matters a lot.
Real time tracking
Have you ever ordered food online which gets packed and a delivery agent is assigned the task of bringing it to you.
You are hungry and a bit annoyed too, to add more to your annoyance, imagine if you can’t live track a delivery agent. In order to get live status, you need to refresh the application every time. Sounds much more than just annoying, right?
This is when Django channels solve this problem.
Live streaming
Watching a movie or sports or video calling someone? No worries, applications built using django channels will be able to handle everything
Advantages of channels
- Transfer of data will be in real time which doesn’t require refresh again and again as a result of which the end user will have a good and smooth experience.
- You are trying to upload a big file with millions of rows, with simple applications you may need to wait longer than expected but with django channels powered applications, you don’t need to wait for the entire file to get uploaded. You can continue with your task while uploading will be in process in the background.
- Since your application can handle those tasks which are asynchronous in nature, you can also scale up your application whenever needed.
Building a chat application using django channels:-
- Create a directory called “channels_demo” and inside that directory create another directory called “chat”.
- Create a virtual environment with the following command.
- Once you have created a virtual environment with the name ‘env’. Activate it with following command
- After activating the virtual environment, install dependencies with the following command. Upon successful installation, you should see these messages.
- Now, go inside chat folder and create a django project with following command
- Navigate into the newly created “chatproject” folder and you should see its contents like this
- Open the folder in vscode. It should open the project in vscode.
- Now open a terminal and activate virtual environment in your vscode terminal
- Test your django application using this command and you should see your server running
- Stop your server and in your settings file of project, insert ‘channels’ inside INSTALLED_APPS like this
- In the same file add following and remove “WSGI_APPLICATION = ‘chatproject.wsgi.application’
- Now run the server with below command,
you should see something like this which means that an ASGI interface is running.
“Starting ASGI/Channels version 3.0.5 development server at https://127.0.0.1:8000/”
- Stop the server and create a new app with following command
- Register your newly created app “chatapp” like you did in step number 10.
- Add the following code in your project settings file. These are channels settings.
- Copy paste the following code in your asgi.py file inside your projects folder
- Create three files “consumers.py”, “urls.py” and “routing.py” inside your chatapp folder.
- Create a folder “template” folder where your manage.py file is, and inside the template folder, make another folder “app”. Make sure to add ‘template’ inside your TEMPLATES in project settings like this.
- Create a template file inside template/app folder called as “index.html”
- Inside your index.html file, write the following piece of code
It is a very basic front end which enables a chatbox along with an input field. In the first two lines of script, you are defining a websocket variable and when it is receiving any message, it is triggering a function which gets the message using “const data = JSON.parse(event.data)” and appends it inside the element that goes by the id chat-log “document.querySelector(‘#chat-log’).value += data.msg + ‘\n’”
element with “chat-message-submit” is also enabled with an onclick event which would get the text of input box and sends it to backend using websocket connection “ws.send(JSON.stringify({‘msg’:message}))” and the it empties the input box.
- Now that you have your index file ready, link it with urls using views. So, inside chatapp/views, insert the following code
- Inside chatapp/urls, add this
Also make sure that you are including your chatapp.urls in your main urls that is chatproject/urls like this
- Now Inside your chatapp/consumers.py file, add this
Sole purpose of this code is to receive clients’ messages and send messages back to the client.
- Websocket_connect handles the connection request.
- websocket_receive handles if there are any messages from the client.
- websocket_disconnect, as the name suggests, is responsible for disconnecting connections.
- chat_message is a method to handle messages that are received.
- Lastly, inside your chatapp/routing.py file, insert this piece of code
from django.urls import path
- Now run the server and visit https://127.0.0.1:8000/ and you should see
- Open another instance of the same application in another tab and try sending a message from another instance, the same message should be reflected in your first instance.
Well done!
DEMO!!
Conclusion:-
It comes into play when we are moving towards building real time applications. Django channels have various use cases in order to increase efficiency of an application.