API Development: A Comprehensive Guide
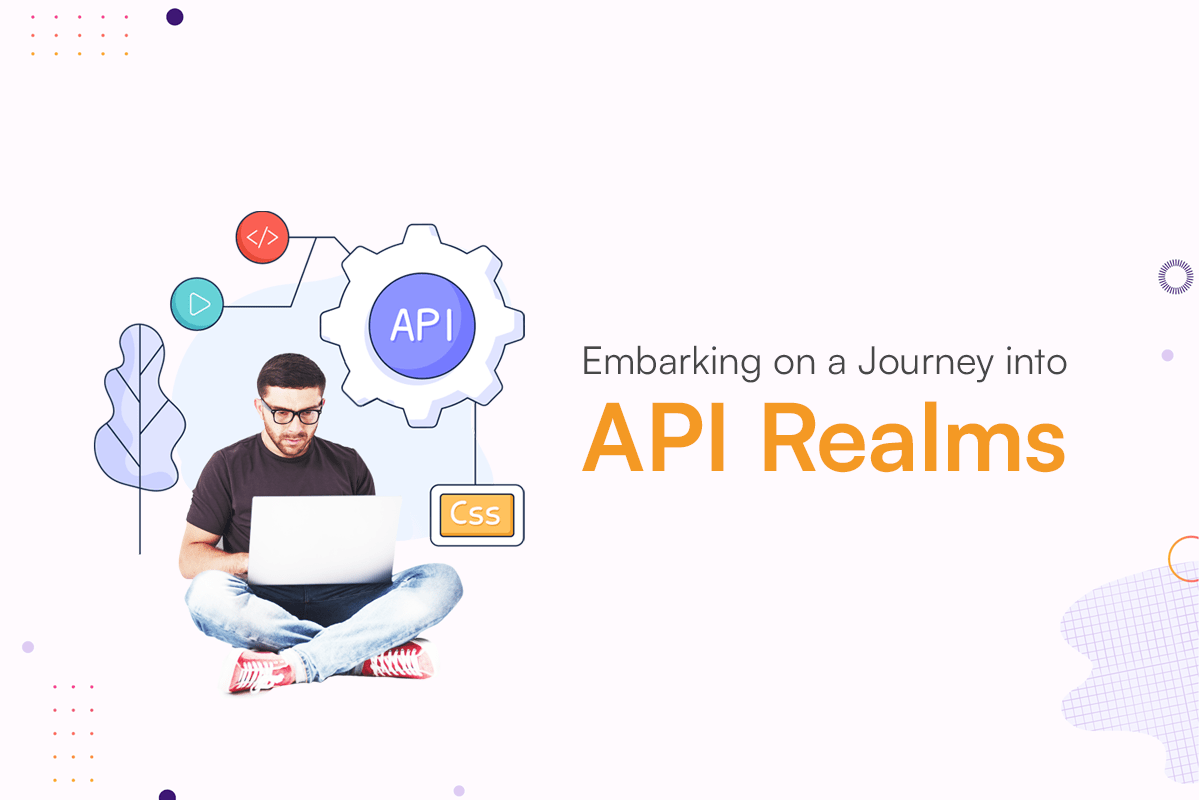
In this modern world of web development, APIs (Application Programming Interfaces) have cemented their place as a tool of prime importance, as these are the basic building blocks that enable seamless communication between different software application. They play an important role in helping developers to program scalable and modular systems, hence enhancing efficiency and user experiences. In part one of our guide, we will dive into the fundamentals of APIs, explore various API types, and shed some light on the principles that encourage effective API designs.
Introduction to APIs
1. Definition and Significance
At core, an API is a collection of protocols and tools that acts as a source of truth for various applications to communicate and interact with each other. Acting as a bridge between different applications, these APIs enable components to connect and exchange data and perform an array of operations, even if they are based on completely different systems. The significance of APIs in modern web development cannot be overplayed enough, as they empower developers to harness the functionalities of existing systems without having to deal with their internal complexities.
2. How do they help in enabling communication?
APIs act as an intermediate, which facilitates effective communication between software applications developed using various programming languages and tools. By abstracting the intricate mechanics underlying interaction with external systems, APIs empower developers to concentrate on crafting the distinctive features of their applications. Consider a weather application that fetches real-time weather data from a remote server using an API. In the absence of APIs, developers would be compelled to navigate the complexities of weather data retrieval, leading to a complex and time-intensive development process.
3. Benefits of APIs
The utilization of APIs confers numerous advantages when constructing software systems:
- Modularity: APIs excel at the concept of modularity by encapsulating different functionalities within separate modules. This heightened modularity enhances the maintainability and reusability of code.
- Scalability: APIs play a crucial role in achieving scalability by extracting the internal workings of software systems. This empowers components to be replaced or upgraded with remarkable ease, without engendering disruptions in the entire system. This inherent scalability proves invaluable as the system evolves over time.
- Rapid Development: The existence of APIs accelerates the development process by facilitating the utilization of pre-built functionalities. This acceleration in development cycles assumes greater significance, particularly when project deadlines are stern.
- Interoperability: APIs function as the cornerstone for seamless integration between distinct applications, enabling them to collaboratively fulfill objectives. This is glaringly evident in scenarios where diverse services, such as payment gateways or social media logins, need to be harmoniously integrated into an application’s framework.
Types of APIs
1. Introduction to API Types
The diverse needs of the digital world and data exchange has given birth to a rich array of API types, each geared towards specific needs. Most used API types include:
- RESTful APIs: A REST API (Representational State Transfer Application Programming Interface) is a set of rules and conventions that allows different applications to communicate and exchange data over the internet. It uses standard HTTP methods to request and manipulate data resources, usually in formats like JSON or XML.
- SOAP APIs: SOAP stands for Simple Object Access Protocol, which is a messaging standard. SOAP utilizes XML data format to declare its request and response messages, relying on XML Schema and other technologies to enforce the structure of its payloads.
- GraphQL: GraphQL is a query language for APIs that allows clients to precisely request the data that they need, reducing over-fetching or under-fetching of information. It offers a more flexible and efficient way to interact with server data compared to traditional REST and SOAP APIs. The standard was developed and open sourced by Facebook and is among one of the most feature packed API standards out in the open.
2. Comparison of API Types
Although they are vastly different, and their use cases depend mostly on the use case of the application, here are some of the key differences that set them apart and usually are the main reason to choose or avoid a particular pattern in a scenario.
Aspect | REST | GraphQL | SOAP |
Data Fetching | Gets fixed data from predefined endpoints using various HTTP methods. | Allows clients to request exactly the data they need in a single query (or request). | Requires pre-defined operations and complex XML payloads for data retrieval. |
Flexibility | Zero to no flexibility as clients receives fixed data structures from endpoints. | Extremely flexible, allowing clients to define their data requirements in queries. | Less flexible due to strict contracts and often requires XML transformation. |
Over-fetching | Very prone to over-fetching, where clients often get more data than needed or must request multiple times to get required data. | Almost eliminates over-fetching by retrieving only the requested data, increasing request efficiency. | May involve over-fetching due to fixed response structures and extensive XML formats. |
Protocol | Uses HTTP methods (GET, POST, PUT, DELETE, PATCH) for communication between client and server. | Uses a single endpoint for querying data, using a POST request with a defined query structure. | Often uses various protocols like HTTP, SMTP, and others, with complicated XML based messaging. |
Complexity | Being one of the most used ways to implement APIs, it has the most resources available and is typically easy to implement initially. Although the complexity can easily get out of hand in more complex situations. | It is hard to initially setup and is not usually recommended for simple cases. But after a certain threshold, the complexity scales down. | SOAP APIs are complex to set up due to WSDL definitions and XML schemas, often suited for enterprise-level applications with a higher learning curve. |
RESTful API Fundamentals
1. Understanding REST Principles and its limitations
RESTful API revolves around the idea of using industry standard HTTP methods to interact with data often referred to as resources, typically represented as URLs just like standard web applications. It fully embraces its statelessness, meaning the server has no clue regarding which client is making a request and no session is either stored or required.
Furthermore, it highlights the importance of utilizing different status codes to convey the result of the requests, helping in effective communication between clients and servers without extensive response messages, as each request is always accompanied with one status code to represent its status, whether success or error or something else.
However, as powerful as it might be, it also comes with its own shortcomings. It usually becomes quite complex as the resource variations grow in number, while also handling common use cases such as file handling, authentication, and authorization. Also, one of the most relatable problems with REST APIs is over or under fetching, where you either get more data than required or less data resulting in multiple requests. Each of these scenarios led to a less efficient request-response cycle.
2. Exploring HTTP Methods
REST uses various HTTP methods to communicate. There are quite a lot of them but two of the most used ones are usually GET and POST, GET, as the name suggests, to retrieve the data, and POST to update/create/delete the data.
But as the complexity of the applications rises, the methods get much more diverse as it is much more concise to use separate methods for related actions. Here are some of the important ones:
- GET: Retrieve data or resources from the server.
- POST: Send data to the server to create a new resource.
- PUT: Update or replace an existing resource on the server.
- DELETE: Remove or delete a resource from the server.
- PATCH: Apply partial changes to an existing resource.
- HEAD: Retrieve only the headers of a resource, without the actual content.
- OPTIONS: Fetch information about the communication options supported by a specific endpoint for a resource.
3. Navigating Status Codes and Response Formats
These are three-digit numbers that are included with the server response to let the client know about the outcome of the request. For example, a 200 indicates a success response, while something like 401 refers to a request which has been parsed by the server, but an error occurred because the client is unauthorized to access it.
There are many status codes, divided into five broad categories:
- 100 -199 (Informational Responses)
- 200 – 299 (Successful Responses)
- 300 – 300 (Redirection Messages)
- 400 – 499 (Client Error Responses)
- 500 – 599 (Server Error Responses)
Apart from that, here are few of the most widely recognizable responses:
- 200 (Success) – When the request is successfully executed
- 400 (Bad Request) – When the server is unable to understand the request
- 401 (Unauthorized Error) – When the client is not authorized to access the requested resource
- 404 (Not Found) – When the requested resource is unavailable, and server does not find anything
- 500 (Internal Server Error) – It means that the server was not able to fulfill the request due to due to some unexpected server state or condition
Response Formats
The preferred response formats of a standard REST API are JSON (JavaScript Object Notation) or XML (Extensible Markup Language).
But more often than not, JSON is used due to its simplicity of parsing, light weightiness and more support for modern programming languages such as JavaScript.
API Design Best Practices
Guidelines for Effective Design
Building a user-friendly and consistent APIs involves adhering to design principles that enhance usability. Albeit there are countless factors that help an API become more effective, here are a few of the important ones. We will dive into the twisted details in the upcoming parts of this guide, but as of now here are the basics:
- Consistent and Clear Naming: Maintain consistent naming conventions for endpoints and parameters to make the API more intuitive to use for developers.
- Proper Use of HTTP Methods: Use appropriate HTTP methods according to their intended actions.
- Error Handling and Responses: Provide meaningful error messages and correct HTTP status codes to guide developers when things go haywire.
- Backward Compatibility: Incorporate versioning from the start to ensure that future changes do not break existing client implementations, maintaining backward compatibility.
- Concise Documentation: Provide clear, thorough documentation that explains how to use endpoints, what to expect in responses, and includes examples. There are tools which can easily help you achieve this without writing everything yourself.
Conclusion
In this first part of our comprehensive guide to API development, we have set our first foot forward on the journey to explore API fundamentals and their significant role in modern web development. We have journeyed through the world of APIs, witnessing how they fill the void between applications, promoting modularity and scalability.
As technology propels us forward, APIs emerge as the kingpin that drives the digital landscape. From enabling simple weather data retrieval to managing complex enterprise applications, APIs have revolutionized the world of software development.