API Optimization: Navigating Advanced Terrain
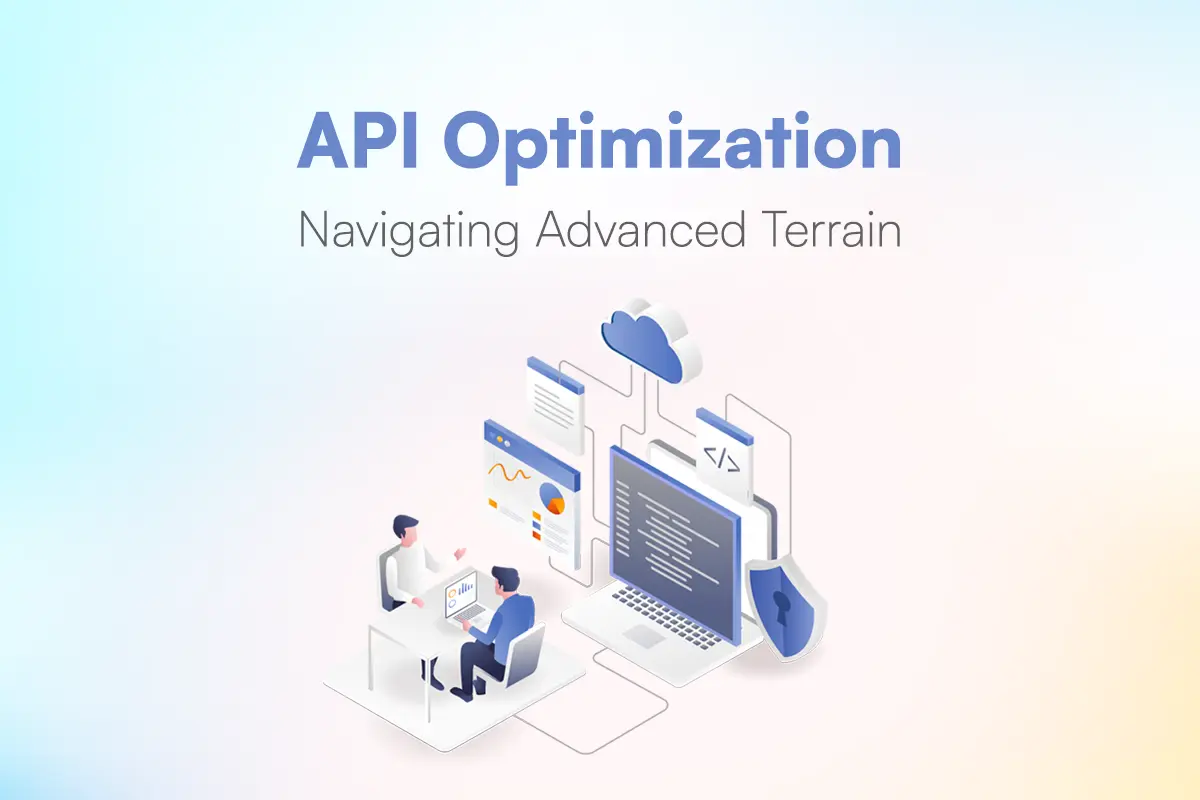
In the previous installments of our API Development series, we dived into the foundational aspects of APIs and explored the intricacies of API development. Now, in the third installment, we embark on an advanced journey, focusing on the critical aspects of managing and optimizing APIs. From handling errors gracefully to predicting future trends, this guide will equip you with essential knowledge to master the art of API management and optimization.
10. Error Handling and Exceptional Cases
In the realm of API development, errors and exceptions are inevitable. How you choose to handle errors can significantly change the user experience and the overall functionality and usability of the application.
1. Techniques for Handling Errors:
a. Meaningful Error Messages:
- Craft error messages that are clear, concise, and easy to understand. Users should immediately grasp what went wrong and why.
For example, messages like “A user_id must be passed to update credentials”, “The email you provided does not correspond to any user” are very good example of good error messages as they immediately tell what is wrong with implementation. Meanwhile examples vague error messages like “An error occurred” are bad as they do not tell anything to the user about what exactly is going wrong.
Also unformatted HTML Errors, full stack traces, database exceptions are also not good error messages including syntax errors.
- Provide specific details about the error. Include information like which API endpoint caused the error, what parameters were invalid, and what actions users can take to resolve the issue.
- Ensure that error messages are user-friendly and avoid too much technical bits. Users, including non-technical ones, should be able to comprehend the message without any confusion.
b. Logging and Monitoring:
- Implement robust logging mechanisms within the API. Log errors on the server-side, capturing relevant information like the endpoint, user, timestamp, and the nature of the error. These logs are invaluable for diagnosing issues during maintenance. There are various tools available in market such as Logstash by Elasticsearch that helps you manage these as well as you can manage your own by writing log files on server using packages like Winston (if you’re using Node)
- Employ real-time monitoring tools to track API usage and performance. Monitoring tools can detect unusual patterns, allowing developers to identify and address errors promptly.
c. Graceful Degradation:
- Implement fallback mechanisms for critical functionalities. If a specific API endpoint fails, the system can revert to alternative methods or cached data to ensure that the user experience is not entirely disrupted.
2. Error Codes and Response Formats:
a. Standardized Error Codes:
- Adhere to a consistent set of error codes across your API. Standardized error codes provide a common language between the API server and its clients, making it easier to identify specific issues.
Also make sure the status of the response is always correct, for example as 200 OK status for an error message could be very misleading for the consumer of the API
- Document the error codes comprehensively in your API documentation. Explain each error code, its potential causes, and the recommended actions that users should take in response to each error.
b. Response Formats:
- As mentioned in earlier blogs, JSON (JavaScript Object Notation) is the most widely used response format due to its simplicity and ease of parsing. Ensure that error responses, like successful responses, are formatted in JSON. This consistency simplifies error handling for client applications regardless of the platform.
Here’s a good example of error and success responses:
Error: https://gist.github.com/srvtechify/7a757efe84b0a55e78e23dab73870d10
Success: https://gist.github.com/srvtechify/84f1ffda0e4302bc54758eea50013dad
- Alongside error codes and messages, consider including additional data in the error response. This could include details such as a unique error ID, a timestamp, or specific parameters causing the error. Such additional data aids developers in diagnosing problems efficiently. These things are especially crucial if you’re making a public API that can be potentially used by many people for whom it may be hard to reach you in times of crisis.
c. Versioning and Backward Compatibility:
- If you introduce changes to error codes or response formats, handle versioning diligently. Clearly communicate the changes, and if possible, maintain backward compatibility with older versions of your API to prevent disruptions for existing users.
- If a new version introduces changes, allow a transition period where both old and new error formats are supported. This transition phase provides developers with time to update their applications gradually.
11. Caching and Performance Optimization
1. Implementing Caching Mechanisms:
a. Understanding Caching:
- Caching Basics: Caching involves storing frequently accessed data temporarily. In API development, caching mechanisms store API responses, reducing the need for the server to regenerate the same response repeatedly. There are great tools that work across multiple languages such as Redish, Varnish, Apache Ignite etc.
- Types of Caching: There are various caching types, including client-side caching (browsers store responses) and server-side caching (servers store responses). Server-side caching is often crucial in API performance optimization.
b. Cache Control Headers:
- Cache-Control Header: Utilize the Cache-Control header in API responses. This header provides directives to both clients and intermediary caching mechanisms, specifying caching policies (e.g., public, private, no-store) and expiration times.
c. Content Delivery Networks (CDNs):
- CDN Integration: Integrate your API with a Content Delivery Network. CDNs cache API responses in multiple locations globally, ensuring users receive responses from the nearest server. This reduces latency and significantly enhances response times for users worldwide. There are many great CDNs available such as AWS CloudFront, Cloudflare CDN, Google Cloud CDN etc.
d. Smart Cache Invalidation:
- Invalidation Strategies: Implement smart cache invalidation strategies. When the underlying data changes, invalidate the cache for the specific resources affected, ensuring users receive up-to-date information. Techniques like cache purging or cache timeouts can be employed.
2. Tips for Optimizing API Response Time:
a. Efficient Database Queries:
- Query Optimization: Optimize database queries to retrieve data efficiently. Use indexes, limit the retrieved columns to necessary ones, and avoid complex joins whenever possible. Well-optimized database queries significantly reduce response time.
b. Data Compression and Minification:
- Compression: Compress API responses using techniques like GZIP compression. Compressed data reduces the amount of data transferred over the network, improving response times, especially for large payloads.
- Minification: Minify JSON, XML, or other response formats. Removing unnecessary whitespace and comments reduces the response size, enhancing data transfer speed.
c. Asynchronous Processing:
- Asynchronous APIs: Implement asynchronous processing for time-consuming tasks such as uploading, photo processing, large calculation etc. Instead of making users wait for a response, accept the request, process it asynchronously, and notify users upon completion. This approach enhances responsiveness and perceived speed. Any task that can take more than a few seconds needs to incorporate some kind of notification mechanism because you cannot expect the user to sit around and wait for API response for 10-20 seconds or even more.
d. Load Balancing and Scalability:
- Load Balancing: Utilize load balancers to distribute incoming API requests across multiple servers. Load balancing ensures that no single server becomes overwhelmed, maintaining consistent response times even during traffic spikes. Load balancing can be achieved directly on your own server with tools such as NGINX but more often than not it is better to offload it to third party services such AWS Elastic Load Balancer.
- Scalability: Design the API to be scalable. Use microservices architecture and cloud-based solutions to scale resources horizontally, adding more servers as demand increases. Scalable architectures prevent performance degradation during traffic spikes. If you’re new to this, you can also leverage serverless platforms to handle scalability automatically, although there are pros and cons to it. We will discuss them separately in another blog. One of the most prominent players in serverless platforms is AWS Lambda that calls a function only when a particular event is fired, such as calling an endpoint.
e. Optimized Code and Algorithm Complexity:
- Code Optimization: Write efficient and optimized code. There are tools that can identify bottlenecks and performance issues. Addressing these issues can lead to significant improvements in API response times.
- Algorithm Complexity: Analyze algorithms that you might be using in your business logic. Choose algorithms with lower time complexity for resource-intensive tasks. Optimizing algorithmic complexity directly impacts the speed of data processing.
12. Version Control and Deprecation
a. Semantic Versioning:
- Semantic Versioning: Adhere to Semantic Versioning principles (major.minor.patch) to clearly communicate changes in your API. Increment the version number based on the impact of the changes:
- Major: Backward-incompatible changes.
- Minor: Backward-compatible new features.
- Patch: Backward-compatible bug fixes.
b. Versioning in Endpoints:
- Include Version in URLs: Incorporate the version number in the API endpoint URLs (e.g., /v1/resource). This makes it explicit which version of the API clients are interacting with and is crucial if you’re willing to support the development of the API for extended years.
c. Backward Compatibility:
- Avoid Breaking Changes: Exercise caution when making changes to existing endpoints. Introduce new endpoints or fields instead of altering existing ones to maintain backward compatibility. If breaking changes are necessary, reserve them for major version updates only.
2. Best Practices for Deprecation:
a. Graceful Deprecation Process:
- Announcement: Announce the deprecation well in advance, preferably at least one version before the removal. Clearly state the deprecated feature, the version it will be removed in, and the alternatives available.
- Transition Period: Allow for a transition period where both the deprecated and the new versions of the API are operational. This provides developers with time to update their applications gradually. Never, not even in a blue moon just decide that you want to remove a feature and directly remove it in a next update, as there could be multiple client-side applications that could be using that feature and no transition period means that those will completely fail to be operational.
b. Clear Documentation:
- Updated Documentation: Update the API documentation to reflect deprecated features and provide information on the recommended alternatives. Ideally leave the complete documentation of the deprecated version under a version’s history section or in a deprecated section.
- Deprecation Notices: Include deprecation notices prominently in the documentation, indicating which endpoints or features are deprecated, and when they will be removed.
c. Sunset Policy:
- Define Sunset Policy: Establish a clear policy for how long deprecated features will be supported after their deprecation. Clearly communicate the timeline to users. After the sunset period, the feature can be safely removed in subsequent versions.
13. Monitoring and Analytics
1. Importance of Monitoring API Usage:
a. Real-time Performance Analysis:
- Identifying Bottlenecks: Monitoring API usage in real-time helps identify bottlenecks and performance issues as they occur. This enables timely interventions to maintain optimal system performance.
- Response Time Analysis: By tracking response times for various API endpoints, developers can pinpoint slow-performing endpoints and optimize them for better user experience.
b. Usage Patterns and Traffic Analysis:
- User Behavior: Monitoring tools provide insights into how users interact with the API. Understanding usage patterns helps in tailoring the API to meet the specific needs and expectations of the user base.
- Traffic Analysis: Analyzing API traffic helps anticipate peak usage times. By scaling resources accordingly, the API can handle high traffic without degradation in performance.
c. Error Detection and Resolution:
- Error Monitoring: Real-time monitoring detects errors and exceptions. Detailed error reports allow developers to identify the root causes, enabling quick resolution and improving the overall reliability of the API.
d. Security and Compliance:
- Security Monitoring: Monitor API requests and responses for security vulnerabilities. Anomalies in API usage patterns can indicate potential security threats, allowing for timely security measures implementation.
- Compliance Checks: Ensure that API usage aligns with regulatory requirements and compliance standards. Monitoring tools can assist in verifying if data transfers and user interactions comply with relevant regulations.
2. Introduction to API Analytics Tools:
a. Data Aggregation and Visualization:
- Aggregating Data: API analytics tools collect and aggregate data related to API requests, responses, errors, and user interactions. Aggregated data provides a comprehensive view of the API’s performance and usage.
A great tool available for this and many other monitoring task is Elastic, providing great info, logging, monitoring and more at the same time.
- Visualization: Visualization tools within analytics platforms present data in intuitive graphs and charts. Visual representations facilitate easier interpretation of complex data, aiding in decision-making processes.
b. User Engagement and Experience:
- User Engagement Metrics: API analytics tools track user engagement metrics such as active users, popular endpoints, and user demographics. These insights help in understanding user preferences and tailoring API functionalities accordingly.
- User Journey Analysis: Advanced analytics tools provide user journey analysis, mapping the sequence of API calls made by individual users. Understanding user journeys aids in optimizing the API workflow for a seamless user experience.
c. Predictive Analytics and Recommendations:
- Predictive Analytics: Some analytics platforms incorporate predictive analytics algorithms. These algorithms analyze historical usage patterns to predict future trends, enabling proactive decision-making and resource allocation.
- Recommendations: API analytics tools can provide recommendations based on user behavior data. Recommendations guide API developers on potential enhancements and optimizations, ensuring continuous improvement.
d. Integrations and Alerting:
- Integration Capabilities: API analytics tools often integrate with various third-party services and platforms. Integrations allow for a holistic view of the system’s performance by incorporating data from multiple sources.
- Alerting Systems: Analytics tools can set up alerting systems. When predefined thresholds are breached (e.g., high error rates), the tool sends notifications, enabling immediate action to address emerging issues.
14. Rate Limiting and Throttling
1. Understanding Rate Limiting:
a. Concept of Rate Limiting:
- Definition: Rate limiting is the process of controlling the number of requests a user or a client can make to an API within a specified time window.
- Importance: Rate limiting is crucial for preventing abuse, ensuring fair usage, and maintaining optimal performance of the API server. It protects against DDoS attacks, brute-force attacks, and ensures equal resource allocation among clients.
b. Throttling:
- Definition: Throttling is a related concept that involves slowing down the rate of requests for users who exceed their allotted limits. Throttling prevents a sudden burst of requests from overwhelming the API server.
- Use Cases: Throttling is particularly useful during traffic spikes, preventing server overload and ensuring a consistent quality of service for all users. It also ensures that no one intentionally tries to overload your servers.
2. Implementing Rate-Limiting Strategies:
a. Identifying User Types:
- User Categories: Categorize users based on their role or subscription level (e.g., free users, paid users, administrators). Apply different rate limits to each category to reflect their usage privileges.
b. Setting Appropriate Limits:
- Granular Rate Limits: Set granular rate limits based on the type of API endpoint and the complexity of requests. For example, reading operations might have higher limits than write operations.
- Consideration of Business Needs: Align rate limits with your business model. For instance, paid subscribers might have higher limits than free users, encouraging users to upgrade for more access.
c. Graceful Error Responses:
- HTTP Status Codes: Return appropriate HTTP status codes (e.g., 429 Too Many Requests) when rate limits are exceeded. Include headers in the response indicating the limit, remaining requests, and the time when the limit will reset.
- Retry-After Header: Use the Retry-After header to inform clients when they can make requests again without hitting the rate limit.
d. Exponential Backoff:
- Exponential Backoff: If a client exceeds the rate limit, implement exponential backoff strategies. Clients wait increasingly longer intervals before retrying, preventing a sudden return of requests when the limit resets.
Conclusion:
In this comprehensive guide’s third installment, we covered the nuanced aspects of managing and optimizing APIs, its past and its future and what to expect in upcoming years. Armed with this knowledge, we are well-equipped to navigate the complexities of API development, ensuring seamless user experiences and future-proof solutions.
Thank you for reading through, and stay tuned for more.