Introduction to Python for Data Analysis with Pandas
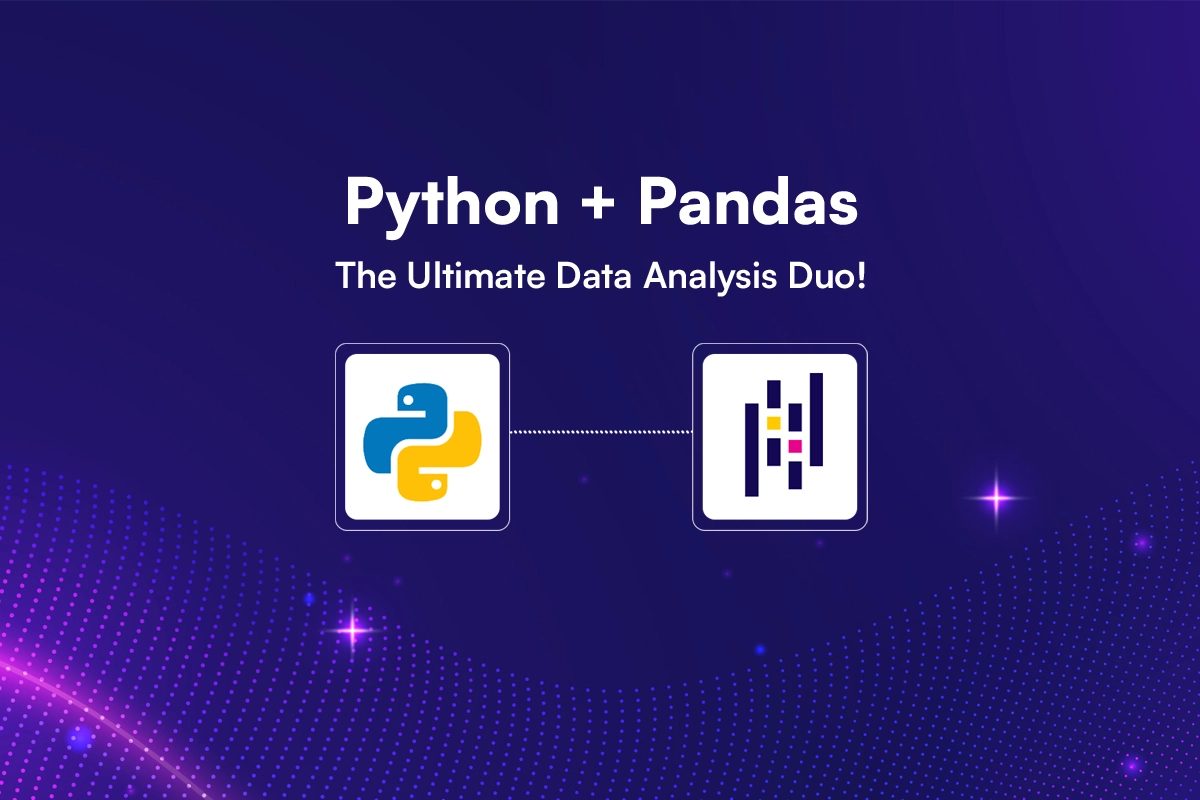
Introduction
Data analysis is a key driver of decision-making in various industries, and Python has become a leading language for this field. Among Python’s powerful libraries, Pandas is essential for its robust and intuitive tools for data manipulation and analysis.
Why Python for Data Analysis?
Python is a top choice for data analysis because:
- It’s simple and beginner-friendly.
- Offers robust libraries like Pandas, NumPy, and Matplotlib.
- Scales from small tasks to large data pipelines.
- Has a vast and active community.
What are Pandas?
Pandas is an open-source Python library designed for structured and tabular data. It simplifies importing, cleaning, transforming, and analyzing data, making it indispensable for data professionals.
Why Use Pandas?
- Simplifies data handling from multiple sources.
- Provides intuitive structures like Series and DataFrames.
- Offers tools for filtering, aggregation, and reshaping data.
- Integrates seamlessly with Python’s data ecosystem.
- Efficiently processes large datasets.
Getting Started with Pandas
1. Installing Pandas
Before diving into data analysis, ensure you have Pandas installed. You can install it via pip:
2. Core Data Structures in Pandas
Pandas is built around two primary data structures: Series and DataFrame.
- Series: A one-dimensional labeled array capable of holding any data type.
- DataFrame: A two-dimensional labeled data structure similar to a spreadsheet or SQL table.
3. Loading Data
Pandas can read data from multiple formats, such as:
- CSV:
- Excel:
- SQL:
Working with DataFrames
- Selecting Data
Think of a DataFrame as a giant table. Often, you’ll need to pick out specific parts of it—like selecting a column or extracting a few rows. Here’s how:
- Single Column Selection
If you want just one column, it’s as simple as: df[‘column_name’] Or, if the column name doesn’t have spaces, you can also do: df.column_name
– Let’s say you need two or more columns. Just pass a list: df[[‘col1’, ‘col2’]].
– You can grab rows using labels or positions:
- Adding and Modifying Data
Imagine you’re working on a dataset, and you realize you need to add a new column or tweak some values. It’s super easy with Pandas.
- Add a new column:
Let’s say we want to predict someone’s age in 10 years:
- Update a specific value:
Maybe someone moved to a different city. Here’s how you can update that:
- Renaming and Deleting Columns
Cleaning up column names or removing unnecessary data is a common task
- Rename columns:
- Delete or remove a column, use this:
Handling Missing Data
In real-world datasets, missing values are everywhere! Here’s how to handle them:
Examples:
- Drop rows with missing values
- Fill missing values:
Sometimes, you don’t want to drop rows. Instead, fill the gaps with a value (like the mean):
Sorting and Filtering
- Sorting
Sorting is like rearranging your table to make it more readable. For example:
- Filtering
Let’s find rows where Age is greater than 30:
Combine multiple conditions with logical operators:
Aggregations and Grouping
- GroupBy Basics
Grouping data is a fundamental operation in data analysis. With Pandas, you can use the groupby method to split data into groups and calculate statistics like sums, means, or counts.
- The agg Method
Want multiple stats in one go? Use agg:
Data Merging and Joining
- Concatenation
Combine DataFrames vertically or horizontally using pd.concat:
- Joins
Perform SQL-style joins (left, right, inner, and outer) using merge:
Advanced Indexing
- Using loc and iloc Accessors
These two methods are your tools for selecting rows and columns in Pandas. Think of loc as the label-based selector and iloc as the position-based selector.
- loc for Label-Based Access
Retrieve rows or columns using labels:
- iloc for Position-Based Access
When you know the exact position, iloc is your friend:
Set and Reset Index
Changing the index can help organize your data for specific use cases.
- Set a Column as Index
- Reset the Index
Bring the default integer index back:
String Operations
- Common String Methods
String operations in Pandas are incredibly useful for cleaning and preprocessing text data. The .str accessor is your gateway to these functionalities.
- Convert to Lowercase
- Remove Whitespace
- Find Substrings
- Filtering with String Methods
Use .str for string-based filtering:
Data Transformation
- The apply Method
The apply method lets you apply custom functions to your DataFrame.
- Apply to a Column
- Apply to Rows
- The melt and pivot Methods
Reshaping your data is often necessary for better analysis.
- Melt
- Melt
Turn wide data into long format:
- Pivot
- Pivot
Turn long data into wide format:
Visualization with Pandas
Pandas has built-in plotting capabilities for quick and easy visualizations (based on Matplotlib).
- Line Plot
- Bar Chart
- Histogram
Conclusion
Pandas is a game-changer for working with data in Python. It makes it super simple to manage, clean, and analyze data, whether you’re selecting specific rows and columns, handling missing values, or visualizing trends. With its easy-to-use tools like DataFrames and Series, you can transform raw data into something meaningful and insightful. So, if you’re diving into data analysis, Pandas is definitely your go-to library!