Integrating Third-Party Services in Your Node.js and React Application
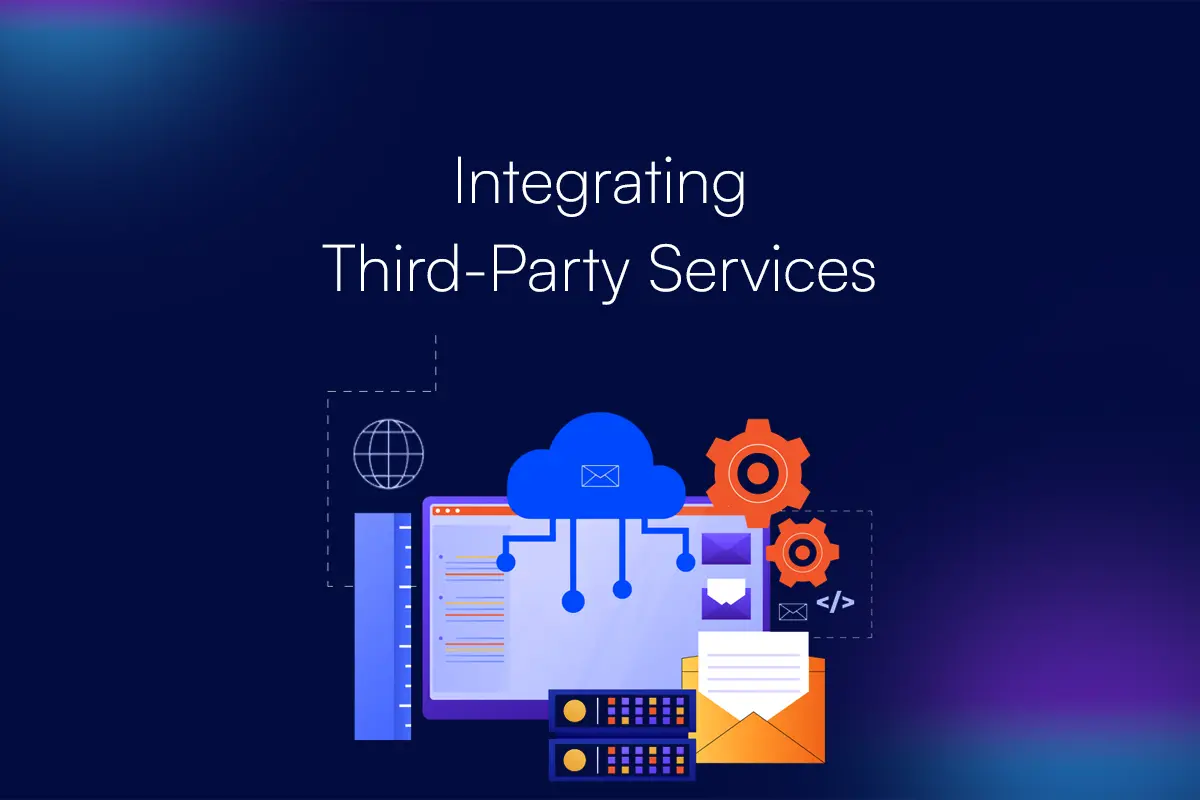
1. Introduction
In the modern web development scenario, integrating third party services using APIs is a common practice. These integrations allow developers to use existing functionalities, such as payment processing, social media sharing, authentication, etc. rather than building these features from scratch. This blog will guide you through the process of integrating third-party services in Node.js and React applications, covering important topics such as APIs, authentication, environment management, and best practices.
2. Understanding APIs
What are APIs?
APIs (Application Programming Interfaces), are sets of rules and protocols that allow different software applications to communicate with each other. They enable developers to access certain functionalities or data from another application, service, or platform. For example, you can use the Google Maps API to embed a map in your application.
3. Integrating Third-Party APIs in Node.js
Making API Calls with Axios/Fetch
In Node.js and other javascript applications, you can make API calls using multiple methods depending on your needs. You can use libraries like Axios or the Fetch API to make those requests. Here is an example of fetching API data with Axios.
Handling API Responses and Errors
Handling responses and errors properly ensures your application behaves reliably. Always check for successful status codes and handle errors gracefully.
Example: Using a Public API
We use a public API, such as the OpenWeatherMap API, to fetch weather data.
4. Integrating Third-Party APIs in React
Making API Calls in React Components
In React, you can make API calls using the Fetch API or Axios directly in your components.
5. Using Third-Party Services for Authentication
Authentication and Authorization
When working with APIs, understanding authentication and authorization is crucial. These processes ensure that the API calls are made by legitimate users or systems.
- API Keys: Simple keys passed in requests to authenticate the caller. They are typically used for basic access control.
- JWT Tokens: JSON Web Tokens are used for more secure authentication, carrying encoded user information that can be verified and trusted.
- OAuth: An open standard for access delegation, commonly used to grant websites or applications limited access to user information without exposing passwords.
Integrating OAuth Providers
OAuth providers like Google, Facebook, and Twitter offer APIs for authentication, allowing users to log in using their existing accounts. These are very beneficial for quick and secure app development as it often wraps very complicated authentication and authorization logics behind easy to use APIs that even relatively new developers can use reliably.
Using Passport.js for Authentication in Node.js
Passport.js is a popular authentication middleware for Node.js. It supports various authentication mechanisms, including OAuth, Google, Facebook etc as its providers to give the developers full control over what kind of authentication experience they want to deliver to their customers.
Here is an example of using Passport.js to implement the famous Login with Google functionality.
Example: Implementing Google Login in a React Application
To implement Google Login in a React application, you can use the Google Sign-In library.
6. Managing Environment Variables
Storing API Keys and Other Sensitive Information
Environment variables are used to store sensitive information like API keys, database URLs, and other credentials securely.
Using dotenv in Node.js
The dotenv package is a popular way to manage environment variables in Node.js., which helps resolve API Keys, and other sensitive variables in a React application.
Using Environment Variables in React apps (Create React App)
In React, environment variables are prefixed with REACT_APP_.
Read: GraphQL vs REST
7. Best Practices
Rate Limiting, Caching, and Optimistic Responses
- Rate Limiting: Implement rate limiting to avoid hitting API rate limits and unpredicted price increase if it is a paid API service.
- Caching: Cache API responses to reduce the number of requests and improve performance and optimise your monthly billing to the minimum.
- Optimistic Responses: Provide immediate feedback to users while the actual API call is being processed, so it gives more app like feeling to the end user for better UX.
Error Handling and Retry
Implement robust error handling and retry mechanisms to handle temporary failures gracefully.
Security Measures
- Use HTTPS to encrypt data that you’re sending in the API calls.
- Validate and sanitize inputs to avoid injection attacks such as SQL injection.
- Rotate API keys periodically to make sure that even if your key is compromised the hackers cannot exploit it for very long.
8. Summary
Integrating third-party services can significantly enhance your Node.js and React applications by leveraging existing functionalities. Understanding APIs, handling authentication, managing environment variables, and following best practices are essential for seamless integration.