Building Scalable APIs: Strategies and Tools for API Development – Part 1
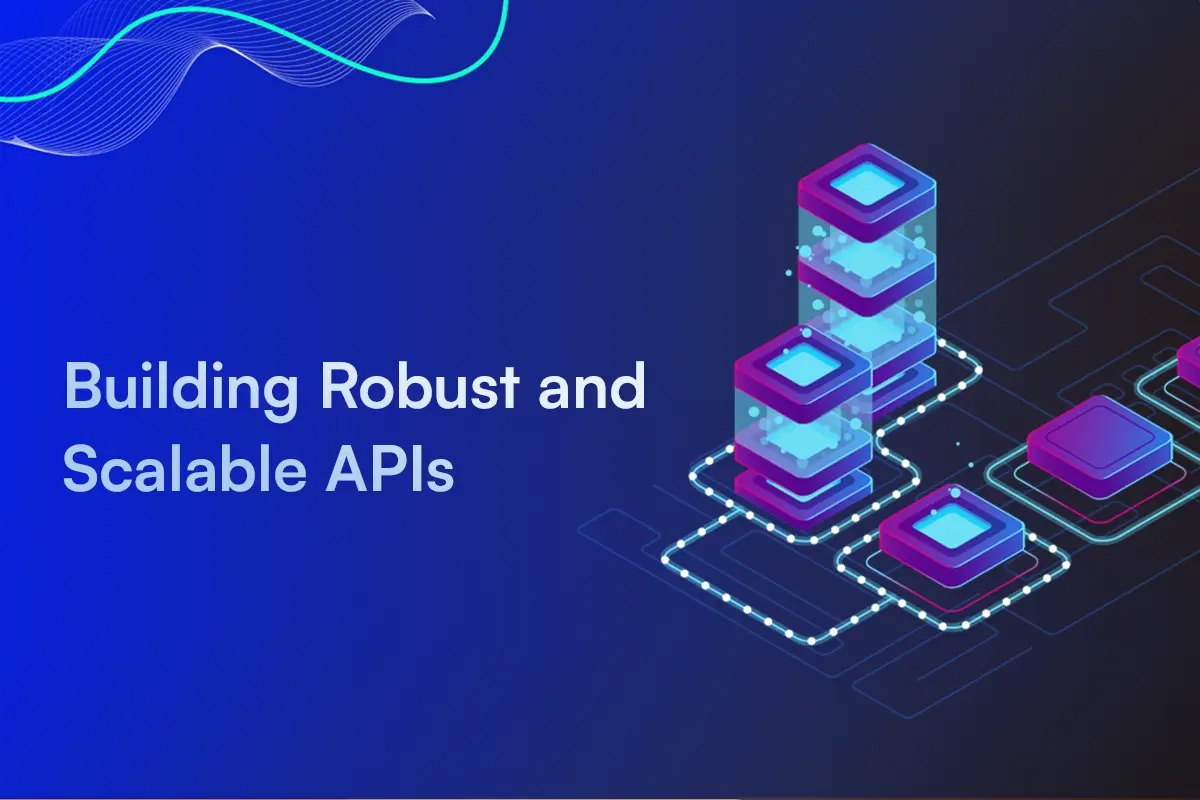
Importance of Scalable APIs
Scalable APIs support business growth by efficiently managing more traffic and higher demands. They provide seamless user experience, boosting customer satisfaction and loyalty. Scalable APIs manage resources efficiently, cutting costs and speeding up the launch of new products and services.
Designing for Scalability
Stateless Architecture
The server doesn’t remember past interactions with a client. Each request is handled on its own, without relying on previous data. This approach makes systems more scalable, reliable, and easier to manage—like a waiter who doesn’t need to remember the last order to serve the next one.
Characteristics
- Request Independence: Each request is independent, with no need for information from the previous requests. The server handles each one as a new transaction.
- Simplified Scalability: Since servers do not need to maintain session state, scaling stateless applications is simpler. Requests can be easily distributed to multiple servers without concern for which server handled previous requests.
- Resilience and Fault Tolerance: Stateless systems are more resilient because they do not rely on session data. If a server fails, another server seamlessly takes over without disrupting the user experience.
- Easier to Cache: Stateless requests are easier to cache as they produce the same response for the same request. This boosts performance and reduces server load.
API Versioning
Implement API versioning to maintain backward compatibility. This lets you update your API without disrupting existing clients, ensuring smooth transitions and continuous improvement.
Benefits
- Backward Compatibility: When an API is updated, clients using older versions might struggle with the changes. Versioning allows those clients to use the older version while new clients or those ready to upgrade can use the latest version.
- Smooth Transition: API versioning offers a clear path for clients to move to newer versions. Developers can support multiple versions simultaneously, allowing clients to upgrade at their own pace.
- Flexibility in Development: Versioning lets developers add new features, improve performance, or fix issues without disrupting existing functionality. It supports continuous improvement while minimizing disruption.
- Clear Communication: Version numbers clearly indicate which version of the API a client is using, making it easier to manage changes and understand the compatibility of different versions.
Strategies:
- URL Versioning: The version number is included in the URL path. Ex. https://api.example.com/v1/users
- Query Parameter Versioning: The version is specified as a query parameter in the URL. ex. https://api.example.com/users?version=1
- Header Versioning: The version is indicated in the HTTP headers. Ex. GET /users Header: API-Version: 1
Rate Limiting
Rate limiting controls how many requests a client can make in a set timeframe. It prevents system overload, protects against abuse, and ensures fair resource allocation. For example, limiting login attempts to 5 per minute can deter brute-force attacks
Strategies:
- Fixed Window Limiting: Requests are limited to a fixed number within a time window, like 1000 requests per hour. Once the limit is reached, any additional requests are blocked until the window resets. Ex. Imagine a movie theater that sells 100 tickets per hour. Once 100 tickets are sold, the counter closes, and no one else can buy a ticket until the next hour begins. If you arrive late and all tickets are sold out, you have to wait for the next hour to try again.
- Sliding Window Limiting: Unlike fixed window limiting, which resets the limit at the end of a time period, sliding window limiting adjusts the limit continuously based on a rolling time window. This ensures a more even distribution of requests over time. Ex. Think of a bus stop where a bus arrives every 15 minutes and can only take 10 passengers at a time. If you arrive and find the bus is full, you’ll have to wait, but as passengers get off at the next stop, seats gradually become available, allowing more people to board at different times instead of all at once.
- Token Bucket: The token bucket algorithm allows each request to consume a token. Tokens are added to the bucket at a steady rate, and when the bucket is empty, further requests are denied until more tokens are available. This approach permits bursts of requests while still enforcing a limit over time. Ex. Imagine you have a toll bridge that issues 10 tokens every minute. Each car crossing the bridge must use one token. If you arrive when all tokens are used up, you’ll have to wait until the bridge issues more tokens before you can cross. However, if tokens are available, you can cross immediately, even if you arrive just after a batch of tokens is issued.
- Leaky Bucket: Requests are added to a bucket that leaks at a steady rate. If too many requests come in at once, the bucket overflows, and excess requests are dropped. This smooths out request rates and handles bursts more effectively. Ex. Picture a bucket with a small hole in the bottom, slowly leaking water. You can pour water (requests) into the bucket, but if you pour too much at once, the excess will overflow and be lost. The water leaking out represents requests being processed steadily, ensuring a consistent flow rather than a flood.
Read: GraphQL vs REST
Efficient Data Management
Database Optimization Techniques
Optimize your database with indexing, query optimization, and partitioning to handle large datasets efficiently. These practices reduce query times and improve overall performance.
Techniques
- Indexing: Indexes speed up data retrieval by allowing the database to find rows more quickly
- Query Optimization: Writing efficient SQL queries reduces the load on the database server and speeds up response times
- Normalization and Denormalization
- Normalization: Reduce redundancy and dependency by organizing data into related tables
- Denormalization: Improve read performance by reducing the need for complex joins
- Partitioning: To divide a large table into smaller, more manageable pieces (partitions), improving performance and manageability
- Use of Stored Procedures: Execute a series of SQL statements with optimized execution plans.
- Database Sharding: Distribute data across multiple database servers, improving scalability and performance.
- Optimizing Hardware Resources: Ensure that the database server has sufficient resources to handle workloads efficiently.
- Database Maintenance: Regular maintenance tasks such as updating statistics, rebuilding indexes, and cleaning up unused data help maintain performance.
- Connection Pooling: Reduce the overhead of establishing database connections by reusing existing connections.
Caching Strategies
Caching is a technique used to improve the performance and efficiency of applications by storing and reusing frequently accessed data. Effective caching strategies can significantly reduce load times, decrease server load, and enhance user experience.
Strategies
- Cache-aside: In a cache-aside strategy, the application first checks if the data is present in the cache. If it’s not, the application loads the data from the database, stores it in the cache, and then returns it to the user. This approach is called “lazy loading” because data is only cached when it’s requested for the first time.
- Write-through Caching: In a write-through strategy, every time data is written to the database, it is also written to the cache simultaneously. This ensures that the cache is always in sync with the database.
- Write-back (Write-behind) Caching: With write-back caching, data is initially written to the cache and the write operation is considered complete. The cache then asynchronously writes the data to the database in the background. This approach can improve write performance but introduces a risk of data loss if the cache fails before the data is written to the database.
- Read-through Caching: In read-through caching, the application interacts only with the cache. If data is requested and not found in the cache, the cache itself retrieves the data from the database, stores it, and returns it to the application. The cache handles both cache misses and subsequent database reads.
- Distributed Caching: Distributed caching involves spreading the cache across multiple servers or nodes to increase cache capacity and availability. This is especially useful for applications that operate at scale, where a single cache server would be insufficient.
- Content Delivery Network (CDN) Caching: CDN caching is a specialized form of caching where static content (like images, videos, and stylesheets) is cached at CDN edge servers closer to the user. This reduces latency and improves load times for users across different geographic locations
Pagination
For endpoints that return large datasets, implement pagination. This approach divides the data into manageable chunks, reducing the amount of data transferred and processed in each request.
Types of Pagination
- Offset-Based Pagination: This technique uses an offset to specify the starting point of the page and a limit to specify the number of records to retrieve
- Cursor-Based Pagination: Cursor-based pagination uses a unique identifier (cursor) from the last record of the current page to fetch the next set of records. Instead of using offsets, the cursor keeps track of the position in the dataset.
- Page-Based Pagination: This method involves specifying a page number and a page size (the number of records per page). The backend calculates the offset based on the page number and size
- Keyset Pagination: Keyset pagination is similar to cursor-based pagination but is particularly efficient for sorted data. It uses a combination of indexed columns to define the “keyset” that the database can use to jump directly to the desired records
- Infinite Scroll: Instead of traditional pagination with numbered pages, infinite scroll loads more data automatically as the user scrolls down the page. This approach is often used in social media feeds and image galleries.
Conclusion
Scalable APIs are essential for managing increasing traffic and maintaining performance in modern applications. Implementing stateless architecture, API versioning, rate limiting, and efficient data management ensures reliability and responsiveness. These practices are vital for delivering seamless service and supporting business growth. In the next article we will learn about Scalability and Resource Management and Performance and Application Design. Stay tuned.