Building Scalable APIs: Strategies and Tools for API Development – Part 2
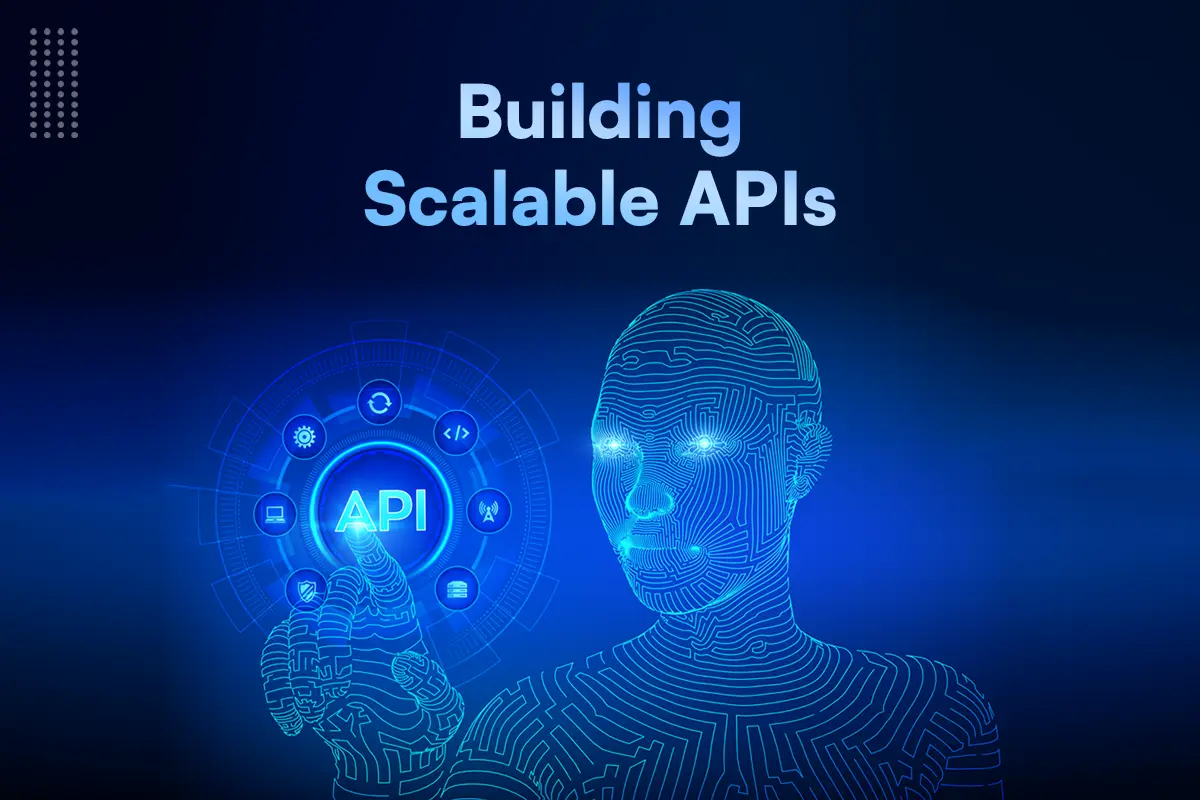
Introduction
In the Previous Article we have learned about Design for Scalability including Stateless architecture, Api Versioning, Rate Limiting and Efficient Data Management Techniques. In this Article we will learn a few more aspects of api scaling like Scalability and Resource Management, Performance and application design.
Scalability and Resource Management
1. Load Balancer
A load balancer is essential in distributed systems and high-traffic environments. It distributes incoming network or application traffic across multiple servers to ensure reliability, performance, and scalability.
Purpose
- Traffic Distribution: Load balancers distribute client requests across multiple servers or backend resources to prevent any single server from becoming overwhelmed with traffic.
- High Availability: Load balancers enhance service availability by rerouting traffic to healthy servers if one server fails or becomes unresponsive.
- Scalability: Load balancers support horizontal scaling by adding or removing servers based on current demand.
- Fault Tolerance: Load balancers detect server failures and redirect traffic to healthy servers, ensuring continuous service.
- SSL Termination: Load balancers can offload SSL/TLS encryption and decryption from backend servers.
- Content Caching: Some load balancers can cache frequently requested content, serving it directly to users without contacting the backend servers.
Types of Load Balancers
- Hardware Load Balancers:
Physical devices dedicated to load balancing, typically used in on-premises data centers. These are Suitable for large enterprises with high traffic volumes that require robust, hardware-based solutions.
- Software Load Balancers:
Software-based solutions that can be installed on standard servers. These are more flexible and scalable than hardware load balancers. Ideal for cloud environments or organizations looking for cost-effective and easily deployable load balancing solutions.
- Layer 4 (Transport Layer) Load Balancers:
Operates at the transport layer (TCP/UDP) and makes routing decisions based on IP address and port number. Suitable for applications that do not require content-based routing, such as email or streaming services.
- Layer 7 (Application Layer) Load Balancers:
Operates at the application layer (HTTP/HTTPS) and makes more complex routing decisions based on the content of the request (e.g., URL, headers). Ideal for web applications where decisions need to be made based on the content of the request, such as directing different URLs to different backend servers.
Benefits
- Improved Availability and Reliability: By distributing traffic across multiple servers, load balancers help keep the application available even if one or more servers fail.
- Scalability: Load balancers simplify adding or removing servers based on traffic demands, enabling efficient scaling of applications
- Enhanced Performance: By optimizing resource usage and minimizing response times, load balancers enhance the overall performance of applications.
- Automatic Failover: If a server fails or becomes unresponsive, the load balancer automatically reroutes traffic to healthy servers, ensuring continuity of service.
- Security: Load balancers can provide an additional layer of security by masking the internal server structure and preventing direct access to backend servers.
2. Horizontal Scaling
Horizontal scaling, or scaling out, increases a system’s capacity and performance by adding more servers or instances. Instead of relying on a single powerful machine, this approach distributes the workload across multiple machines, helping manage higher traffic, improve performance, and ensure high availability.
Benefits
- Improved Performance:
Distributes the workload across multiple instances, allowing the system to handle larger volumes of requests or data more efficiently and improving overall performance.
- Enhanced Reliability:
Provides redundancy by running multiple instances independently. If one instance fails, others can continue handling the workload, increasing reliability and minimizing downtime.
- Scalability:
Offers flexible and granular scaling by adding or removing instances based on demand. This lets the system grow and adapt to varying workloads without major changes.
- Cost Efficiency:
In cloud environments, horizontal scaling is cost-effective because you only pay for the resources you use. You can scale out during peak times and scale back in when demand decreases, optimizing costs.
- Flexibility:
Supports various architectures and applications, from web servers to microservices, allowing systems to adapt to changing demands and technological advancements without major redesigns.
3. Auto Scaling
Auto Scaling is a cloud computing feature that automatically adjusts the number of computing resources available to an application based on real-time demand and predefined criteria. This dynamic adjustment helps ensure that applications have the right amount of resources to handle varying loads efficiently without manual intervention.
Purpose
- Dynamic Resource Management: Auto Scaling automatically adjusts the number of resources (like servers or virtual machines) based on current demand. It ensures applications handle varying loads effectively by scaling up when demand increases and scaling down when it decreases.
- Cost Efficiency: By adjusting resources based on demand, Auto Scaling helps optimize costs. You pay only for the resources you use, reducing waste and avoiding over-provisioning.
- Performance Optimization: Auto Scaling maintains application performance by adding resources during peak times to handle increased traffic or processing needs, and reducing resources during low demand to avoid underutilization.
Benefits
- Improved Availability: Automatically adjusting resources helps maintain high availability and performance, even during sudden spikes in traffic or workload. It ensures the system can handle changes in demand without manual intervention.
- Cost Savings: Auto Scaling helps control costs by automatically scaling down resources when they’re no longer needed. This prevents over-provisioning and reduces expenses for unused resources.
- Enhanced Performance: Ensures optimal performance by providing sufficient resources during high demand periods, which helps avoid slowdowns or service interruptions. It maintains a balance between performance and cost.
- Reduced Manual Intervention: Automates the scaling process, reducing the need for manual monitoring and adjustments. This simplifies resource management and lets teams focus on other critical tasks.
- Adaptability: Adapts to changing workloads and demand patterns in real-time. Whether handling traffic spikes or quiet periods, Auto Scaling keeps the application responsive and efficient.
- Resilience: Enhances application resilience by automatically adjusting resources to maintain performance and availability. It helps the system handle failures or disruptions by scaling resources up or down as needed.
Performance and Application Design
1. Microservices Architecture
Break down your API into smaller, independent services that can be scaled individually. Microservices allow you to manage different parts of your application separately, providing greater flexibility and scalability.
Characteristic
- Independent Deployment
Each microservice can be deployed independently, allowing for continuous delivery and deployment. Updates or fixes to one service can be made without redeploying the entire application.
- Service Autonomy
Microservices are self-contained and handle specific business functions. Each service manages its own data, logic, and state, promoting loose coupling between services.
- Scalability
Microservices can be scaled independently based on demand. For example, if one service experiences high load, it can be scaled up without impacting other parts of the system
- Technology Diversity
Teams can select the best technology stack for each microservice based on its specific needs. This enables the use of different programming languages, databases, and frameworks within the same application.
- API-Based Communication
Microservices communicate through well-defined APIs, using REST, gRPC, or messaging protocols like RabbitMQ or Kafka. This API-driven communication ensures clear interaction contracts between services.
2. Asynchronous Processing
Asynchronous processing lets tasks run independently of the main program flow, allowing the system to continue working while waiting for time consuming operations like network requests or file handling to complete.
Characteristics
- Non-Blocking Operations:
In asynchronous processing, tasks that require waiting for resources (like reading a file or making a network request) don’t block the main program. Instead, the program continues running other tasks while waiting for the operation to complete.
- Concurrency:
Asynchronous processing enables concurrent execution of multiple tasks, allowing the system to handle several tasks at once without waiting for each to finish in sequence.
- Event-Driven Execution:
Tasks in asynchronous processing are often event-driven, meaning they are executed or resumed in response to specific events, such as the completion of a network request.
- Callback Functions and Promises:
Asynchronous operations typically involve mechanisms like callback functions, promises, or async/await syntax to manage the flow of execution and handle the results of asynchronous tasks.
Benefits
- Improved Performance:
By running tasks concurrently, asynchronous processing makes better use of system resources, leading to faster execution times, especially for I/O operations.
- Enhanced User Experience:
In user-facing applications, asynchronous processing prevents the interface from freezing while tasks are completed, resulting in a smoother and more responsive experience.
- Scalability:
Asynchronous systems can handle more tasks simultaneously, making them more scalable. This is crucial for web servers or services that need to manage many client requests at once.
- Resource Efficiency:
Asynchronous processing reduces idle time for the CPU and other resources by allowing tasks to execute while waiting for other operations to complete, leading to more efficient use of system resources.
Conclusion
In this article, we delved into key aspects of API scalability, focusing on resource management and performance optimization. Understanding load balancers, horizontal scaling, and auto scaling is crucial for maintaining high availability and efficient resource use. Adopting microservices architecture and asynchronous processing further enhances flexibility and scalability. Together, these strategies ensure your applications can handle increasing demands while remaining resilient and cost-effective.In the next article we will learn about Monitoring and Logging, Performance and Security, Compliance. Stay tuned.