Understanding RESTful API Development: Best Practices and Common Pitfalls
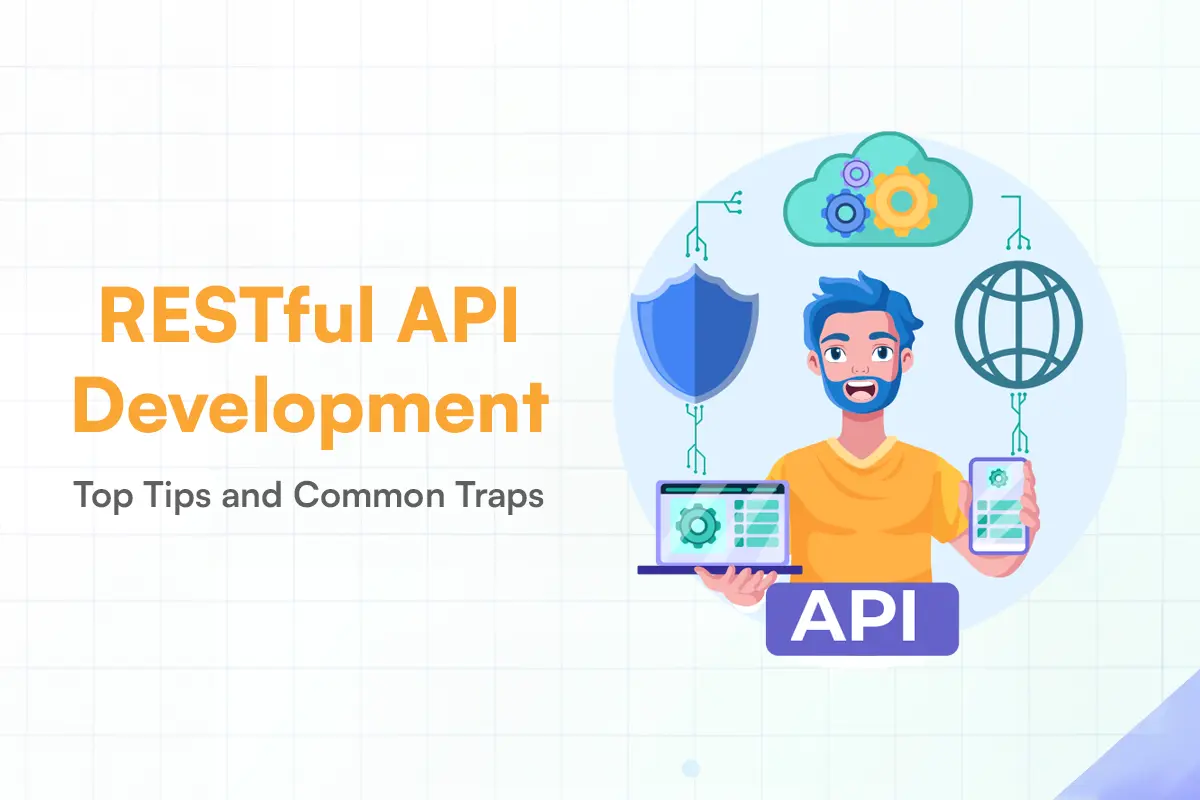
In our previous blogs on API development, we started our journey into the fundamentals of APIs, explored various API types, and discussed essential principles. If you haven’t read them yet, you can find them here.
In this blog, we delve deeper into RESTful API development, focusing on the principles of REST, best practices for designing RESTful APIs, and common mistakes to avoid.
Principles of REST
REST (Representational State Transfer) is an architectural style for designing networked applications. It relies on a stateless, client-server communication protocol, typically HTTP. Here are the core principles of REST:
- Statelessness: Each request from a client to a server must contain all the information needed to understand and process the request. The server does not store any state about the client session.
- Client-Server Architecture: The client and server are separate entities, allowing them to evolve independently. The client handles the user interface and user experience, while the server manages data storage and business logic.
- Cacheability: Responses from the server must be explicitly marked as cacheable or non-cacheable to improve performance.
- Uniform Interface: A consistent interface simplifies and decouples the architecture. It includes identifying resources, manipulating resources through representations, self-descriptive messages, and hypermedia as the engine of application state (HATEOAS).
- Layered System: The architecture can have multiple layers, such as security, load balancing, and data storage, improving scalability and flexibility.
- Code on Demand (optional): Servers can extend client functionality by transmitting executable code (e.g., JavaScript).
Best Practices for Designing RESTful APIs
Use Nouns for Endpoints: Endpoints should represent resources (e.g., /users, /orders) rather than actions (/getUser, /createOrder). Use HTTP methods (GET, POST, PUT, DELETE) to specify actions.
Example:
- Consistent Naming Conventions: Use consistent naming conventions for endpoints and parameters to ensure clarity and predictability. Stick to lowercase letters and hyphens to separate words (e.g., /user-profiles).
Example:
http
Copy code
GET https://api.example.com/v1/user-profiles - Versioning: Implement versioning to manage changes and ensure backward compatibility. Use URL versioning (e.g., /v1/users) or header versioning.
Example:
http
Copy code
GET https://api.example.com/v1/users - Use HTTP Status Codes: Leverage standard HTTP status codes to indicate the result of a request. For example, use 200 OK for success, 201 Created for resource creation, 400 Bad Request for client errors, and 500 Internal Server Error for server issues.
Example:
json
Copy code
- Handle Errors Gracefully: Provide meaningful error messages with relevant status codes. Include details about what went wrong and how to fix it.
Example:
- Pagination and Filtering: Implement pagination and filtering for endpoints that return large datasets. Use query parameters like page, pageSize, sort, and filter to allow clients to customize responses.
Example:
- Documentation: Provide clear and comprehensive API documentation using tools like OpenAPI (Swagger) or Postman. Include examples of requests and responses, authentication methods, and error handling.
- Security: Implement robust authentication and authorization mechanisms. Use HTTPS to encrypt data in transit. Validate and sanitize all input to prevent security vulnerabilities like SQL injection and XSS.
Common Pitfalls to Avoid
- Ignoring Versioning: Failing to version APIs can lead to breaking changes for clients. Always version your APIs to allow for incremental improvements and backward compatibility.
- Overloading Endpoints: Avoid creating overly complex endpoints that handle multiple actions. Stick to the single responsibility principle by designing endpoints to perform one specific action.
Example:
- Inconsistent Error Handling: Inconsistent or vague error messages make it difficult for clients to debug issues. Provide clear and consistent error messages with appropriate status codes.
- Ignoring Caching: Not leveraging caching can lead to performance bottlenecks. Use HTTP caching headers to enable clients and intermediaries to cache responses.
- Lack of Documentation: Poor or missing documentation can lead to confusion and misuse of your API. Invest time in creating detailed and up-to-date documentation.
- Inadequate Security: Weak security practices can expose your API to attacks. Ensure proper authentication, authorization, and data validation mechanisms are in place.
By following these best practices and avoiding common pitfalls, you can design RESTful APIs that are robust, scalable, and easy to use. In our next blog, we will explore advanced topics in API development and management, so stay tuned!