A Comprehensive Guide to Creating Dynamic Surveys with React JS
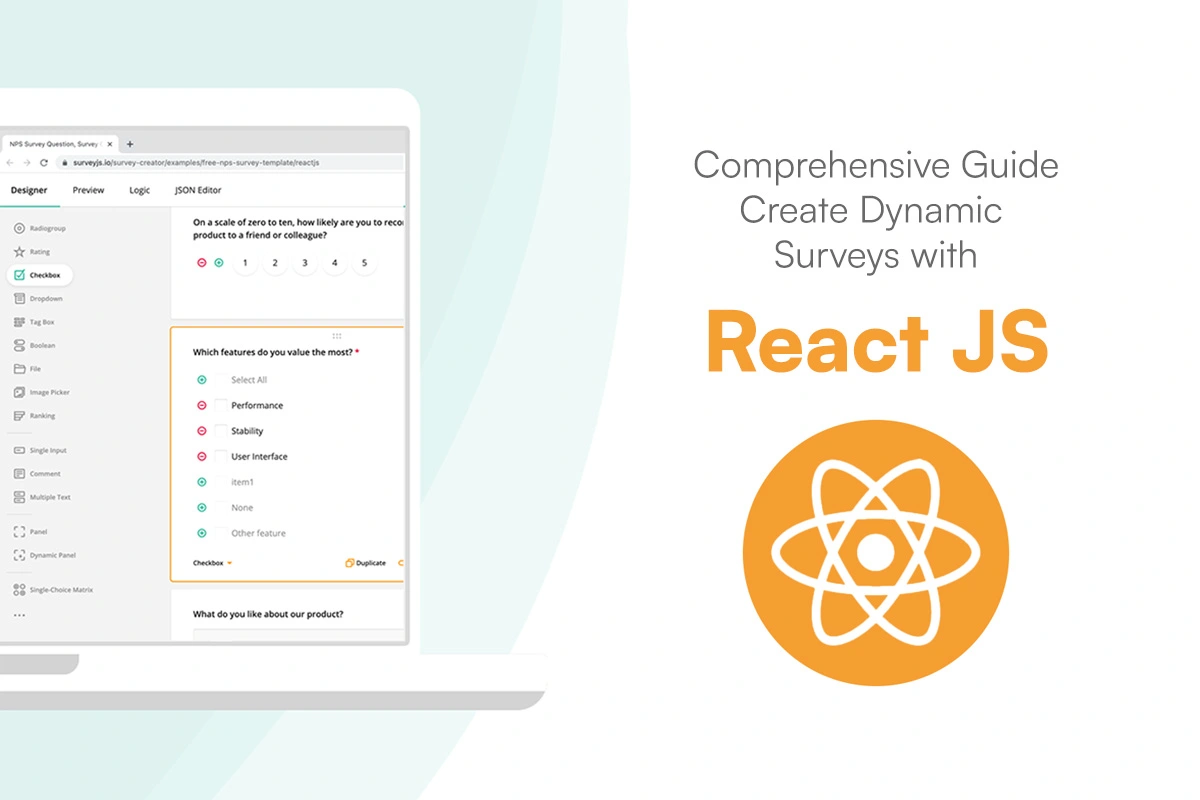
Introduction
Survey JS is a free and open-source MIT-licensed JavaScript library that let you design dynamic, data-driven, multi-language survey forms and run them in your web applications using a variety of front-end technologies.
The SurveyJS Form Library empowers you to effortlessly design, implement, and manage dynamic forms and surveys that seamlessly integrate into your JavaScript-based applications. With this tool, you can design dynamic questionnaires and seamlessly embed them within your application’s user interface. It provides a user-friendly interface for creating a wide array of question types, from simple to complex, while offering customization options, branching logic, and real-time previews. With SurveyJS, you can seamlessly embed surveys into websites, applications, or standalone pages, facilitating data collection, user engagement, and informed decision-making. Whether for market research, feedback collection, or academic studies, SurveyJS simplifies the process of creating interactive and impactful surveys, making it a valuable tool for a range of industries and purposes.
Form Library lets you load dynamic, multi-language, on-page forms and have them run on any of your favourite front-end platforms.
Why SurveyJS Form Library? (Features)
- Create interactive forms, surveys, and quizzes within your JavaScript-based application.
- Drag-and-Drop UI : No-code WYSIWYG form builder to auto generate a form data model—a schema written in JSON. The intuitive drag-and-drop interface allows you to quickly add a variety of question types, including multiple-choice, open-ended, rating scales, and more.
- Native support for multiple platforms like React, Vue, Angular, and Knockout.
- Input Field Variety: 20+ built-in question types and support for custom question types.
- Multi Language Support : Community-supported UI localization to 50+ languages.
- Conditional Logic: Tailor the survey experience based on respondents’ answers using conditional logic. Show or hide questions based on previous responses, enabling a dynamic and personalized interaction that enhances engagement and accuracy.
- Answer validation.
- Modern, customizable UI themes.
- TypeScript support.
- Backend Freedom: Compatibility with any server + database combination. Survey results can be stored in any database.
- Versatile Question Types: Choose from an extensive range of question types to create surveys that suit your objectives. From simple single-choice questions to complex matrix-style queries, SurveyJS.io offers the flexibility to capture the exact information you require.
- Multi-Platform Compatibility: Surveys created with SurveyJS.io are fully responsive and can be accessed seamlessly across various devices, including desktops, tablets, and smartphones. This ensures a consistent experience for respondents regardless of their chosen platform.
- For more information, please click https://surveyjs.io/features.
Add a Survey to a React Application : Get Started in a Few Simple Steps
This step-by-step tutorial will help you to get started with the SurveyJS Form Library in a React application. To incorporate a survey into your React application, adhere to the following guidelines:
1) Choose your front end as React JS & create a project by running npx create-react-app
2) Install the survey-react-ui npm Package: npm install survey-react-ui
The SurveyJS Form Library for React comprises two distinct npm packages: survey-core, which contains platform-agnostic code, and survey-react-ui, which houses the rendering components. Run the following command to install survey-react-ui. The survey-core package will be installed automatically because it is listed in survey-react-ui dependencies.
3) Configure Styles
SurveyJS includes two pre-built UI themes, namely Modern and Default V2, which are visually represented below.
Open the React component in which your survey will be and import a style sheet that implements the required theme.
Default V2 theme : import ‘survey-core/defaultV2.min.css’;
Modern theme: import ‘survey-core/modern.min.css’;
For more information about SurveyJS themes, refer to the following help topic: Themes & Styles.(https://surveyjs.io/form-library/documentation/manage-default-themes-and-styles).
4) Create survey by clicking here: https://surveyjs.io/create-free-survey
- Here you can design your survey details and generate a json file for that.
- Preview survey design using design tab.
- You can customize your theme using the theme tab.
- json data you can find in the Json editor tab.
5) Create JSON data
Creating JSON data for a survey using the SurveyJS online survey builder is a straightforward process. Here’s a brief description:
- Visit the SurveyJS Online Survey Builder: Go to https://surveyjs.io/create-free-survey to access the online survey builder.
- Create a New Survey: Click on the “Create a Free Survey” button to start a new survey project.
- Add Survey Questions: Use the survey builder’s user-friendly interface to add questions to your survey. You can choose from various question types like multiple choice, text input, rating scales, and more. Enter your questions, provide answer choices, and set any additional properties or conditions.
- Export JSON Data: Once you’re satisfied with your survey, you can export the survey data in JSON format. Look for an option like “Export” or “Download” within the survey builder. The JSON file will contain all the questions, choices, and settings you configured in the builder.
- Save and Use the JSON Data: Save the JSON data to your computer or copy it to your clipboard. You can now use this JSON data within your React application to integrate the SurveyJS survey.
You can find sample json data here: https://pastebin.com/clone/UuqS5L15
6) Create a Model
A model defines the structure and elements of your survey. The most basic survey model consists of one or more questions without any layout customizations.
As an illustration, the subsequent model schema (JSON objects) defines two text-based questions, each possessing both a title and a designated name. Titles are exhibited on the user interface, while the names serve as identifiers for these questions within the code.
To instantiate a model, pass the model schema to the Model constructor.
The model instance will be later used to render the survey.
Please refer given link for more information: https://surveyjs.io/form-library/documentation/get-started-react#create-a-model
7) Render the Survey
To render a survey, begin by importing the Survey component. Afterward, include it within your JSX code. Then, you’ll need to supply the model instance you previously constructed to the component’s “model” attribute. This approach ensures that your survey is presented and behaves as intended within your application.
If you replicate the code correctly, you should see the following survey as per given below :
8) Collect submitted form data
Upon the completion of a survey by a respondent, the gathered results become accessible within the onComplete event handler.
The “onComplete” event handler in SurveyJS is a pivotal feature that allows you to take immediate action when a respondent finishes a survey. It provides you with access to the survey data, enabling you to process, store, and analyze the responses effectively.
please refer given link for more information: https://surveyjs.io/form-library/documentation/get-started-react#handle-survey-completion
9) Get data from API and fill up in form dropdown
Please refer below steps to fetch data from an API in a SurveyJS survey within a React application, you can follow these general steps:
- Create a React Component: Start by creating a React component where you want to embed your SurveyJS survey. You can use the useState hook to manage the API data.
- Fetch Data from the API: Use the fetch or a library like Axios to make an API request and retrieve the data. Typically, this is done in a useEffect hook to ensure it happens after the component has mounted.
- Parse the API Response: Once you receive the data from the API, parse it into the format that SurveyJS expects for your dropdown question. This usually involves converting it into an array of options with value and text properties.
- Set State with API Data: Update the state of your component with the API data. This state will be used to populate the dropdown options in your SurveyJS survey.
- Configure the SurveyJS Survey: Finally, configure your SurveyJS survey to use the state data to populate the dropdown question dynamically.
Update your survey Json with fetch API data:
As per above code you will get state data from API to form as given in SS below:
Customize Theme:
When it comes to creating engaging and visually appealing surveys, the right design can make all the difference. Enter SurveyJS and its powerful Theme Editor, a tool that allows you to harness the magic of CSS variables to customize colors, fonts, sizes, and more, giving your surveys a unique and captivating appearance.
Please refer below steps:
- Go to https://surveyjs.io/create-free-survey & select Theme tab.
- At right side Theme Setting you can customize theme & export json by clicking download button there:
To deck your survey in a unique digital attire, take the JSON masterpiece you’ve downloaded and gracefully hand it over to the SurveyModel’s applyTheme() function. Watch as your survey transforms into a bespoke masterpiece, reflecting your distinct style and personality.
Please refer given link for more information: https://surveyjs.io/form-library/documentation/manage-default-themes-and-styles#create-a-custom-theme
Here you will get a customized theme.
Conclusion:
In conclusion, integrating SurveyJS into your ReactJS applications can greatly enhance your ability to gather valuable insights and feedback from users in an interactive and user-friendly manner. With its comprehensive set of features and customizable options, SurveyJS empowers developers to design and deploy surveys, forms, and quizzes effortlessly.
Whether you’re building customer feedback forms, employee satisfaction surveys, or educational quizzes, SurveyJS simplifies the process by providing a versatile toolkit that streamlines the creation and management of dynamic surveys. By combining React’s component-based architecture with SurveyJS’s intuitive JSON format, developers can seamlessly embed surveys into their applications and ensure a seamless user experience.
In this blog post, we’ve explored the key steps to get started with SurveyJS in a ReactJS project, from installation to defining survey questions, handling responses, and customizing the appearance. By following these steps and referring to the official documentation and resources, you’re well-equipped to harness the power of SurveyJS for your own applications.
As you embark on your journey to creating engaging and data-driven experiences for your users, remember that SurveyJS not only enhances user engagement but also provides you with valuable insights that can drive informed decisions and improvements. So go ahead, unlock the potential of surveys and forms with SurveyJS, and elevate the interactivity and intelligence of your ReactJS applications today!