State Management in React: Context API and Redux
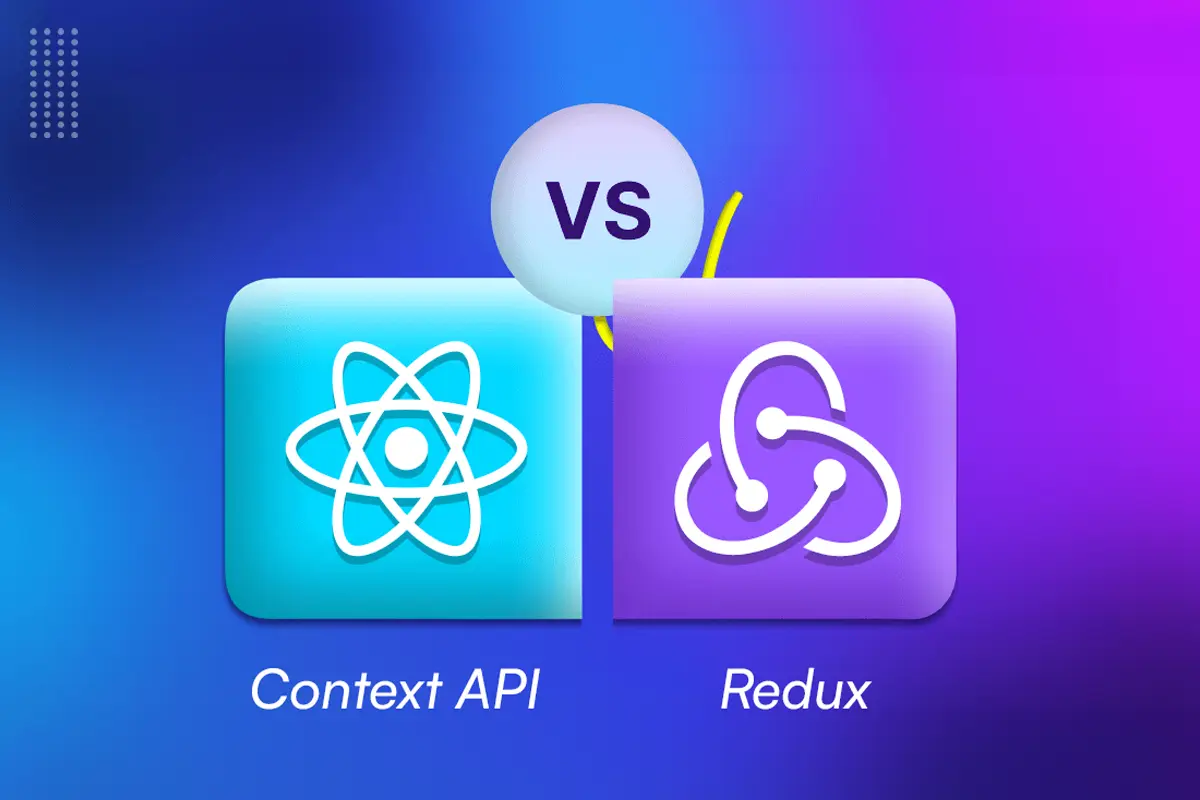
What is State Management?
State management refers to the process of managing the state of an application. The state is a piece of an object that holds the data that can change over time and affect the looks(rendering) and behavior of the application.
State Management in React
React allows us to create dynamic web applications. Managing state in React can be a bit tricky, especially if you’re new to it, as it has multiple approaches to achieve state management rather than one solution. It provides built-in features like the “useState” hook for managing state at the component level and the Context API for global state management. Additionally, there is a pool of external libraries like Redux to handle more complex state management needs.
Why do we need state management solutions?
State management solutions are essential for addressing issues like prop drilling and data sharing between components. Prop drilling occurs when you need to pass state through many levels of components, which can make the codebase hard to maintain and understand.
Additionally, when multiple components need to share data, managing this state without a proper solution can become cumbersome and lead to inconsistencies. Effective state management solutions help to centralize and streamline state handling, making the application more predictable and easier to debug.
Importance of Choosing the Right State Management Solution
Choosing the right state management solution is crucial for the scalability, maintainability, and performance of the application. Now, the right solution can depend on the complexity of the application, your expertise, and specific use cases.
Context API
What is the Context API?
The Context API in React enables you to share data across multiple components without manually passing props down through each level of the component tree.
When to Use Context API
The Context API is best suited for scenarios where you need to share state across a small to medium-sized application (sometimes in more complex scenarios as well). It is more suitable for storing pieces of data like themes, localization, authentication state etc. that doesn’t change frequently.
Advantages & Disadvantages of Context API
Advantages:
- Easy to implement and use
- Already built into React
- Simple syntax with less boilerplate
Disadvantages:
- It can lead to performance issues if not used correctly
- Not ideal for managing complex states like analytics, user data etc.
Redux
What is Redux?
Redux is an open-source JavaScript library for managing and centralizing application state. It is one of the, if not the most popular library for managing complex state in React applications of all sizes.
Core Concepts of Redux
- Store: An object stored at a central location where the state of the application is kept.
- Actions: Actions are plain JavaScript object that contains information (called payload).
- Reducers: Pure JavaScript functions that take the current state and an action and return a new object that is then stored.
- Dispatch: A function used to send actions to the store so that it can update the state accordingly.
- Immutability: The store object in Redux is never updated, it is only ever replaced by a new state object.
When to Use Redux
Redux is mostly used and best suited for large to medium scale applications with complex state and data needs. It’s particularly useful when you have a lot of state changes, data fetching and multiple components that need to share data horizontally and vertically, which means sharing data across sibling components (horizontally) and sharing data between parent and child components (vertically).
Advantages & Disadvantages of Redux
Advantages:
- Predictable state management
- Makes sharing the same data across multiple components a hassle free task once implemented
- Easier debugging with time-travel capabilities
- Largest ecosystem of supported plugins and community support
Disadvantages:
- Harder to grasp concepts when initially getting started
- More boilerplate code, hence can be an overkill for simple application
- Wrong configurations and handling can lead to hard to fix performance issues
Comparing Both Redux and Context API
Scalability and Performance
- Context API: Suitable for small to medium-sized applications. Although you might face performance issues with states that are too frequently changed.
- Redux: Better suited for large enterprise grade applications with complex state. Offers much better performance with increasingly complex state management needs such as analytical data etc.
Boilerplate and Code Complexity
- Context API: Less boilerplate code, quite easy to set up, even for people new to React.
- Redux: More boilerplate, more complex setup. Can lead to a more complex codebase but offers better organization once the entire process is setup.
Library Ecosystem and Support
- Context API: As a part of React, mostly no support for external libraries or plugins.
- Redux: Very large ecosystem with numerous middleware and tools such as Redux Toolkit, Thunk, Saga, Persistence etc.
Use Cases and Examples
Simple State Management with Context API
An application that needs to manage authenticated states, locales, themes, user preferences etc. the Context API can be a straightforward and better solution, as these do not change often and the data stored is relatively pretty simple as well.
Here is a simple example for storing authentication state using Context API:
// store.js
// Home.js
Complex State Management with Redux
An application with complex states, data fetching or frequent updates often are more suitable and performant with React. Apps like a e-commerce shopping carts, dashboards, analytic monitors, CRMs, CMSs, Redux provides a much more robust solution:
Here is a simple example for managing product data in a card:
// actions.js
// reducers.js
// store.js
// app.js
// Cart.js
Best Practices
Choosing Between Context API and Redux
- Context API: Choose for simpler states, which have less frequent state changes.
- Redux: Choose for complex, frequently changing state with a need to be performant and efficient with robust debugging tools both in browser and while developing.
Best Implementation Techniques
- Modularize your Redux code by separating actions, reducers, and store configuration.
- Make sure to never update the state directly, but always dispatch actions to do so.
- Always use middlewares like thunk or saga to handle asynchronous functions (like API calls) rather than manually creating requests, errors and loading phases.
Avoiding Common Mistakes
- Avoid using Context API for complex states, which can lead to performance issues and unpredictable render cycles.
- Keep Redux actions and reducers pure and simple.
- If the data that is being stored in the component does not need to be accessed anywhere else or only to the immediate child component, then it is better to use component state (useState) instead of using global state
Summary
State management is a very critical aspect of building robust React applications. The Context API offers a lightweight solution for simple state management needs, while Redux provides a more scalable and predictable approach for complex state management.