Redux: Simplifying State Management in React Web Applications
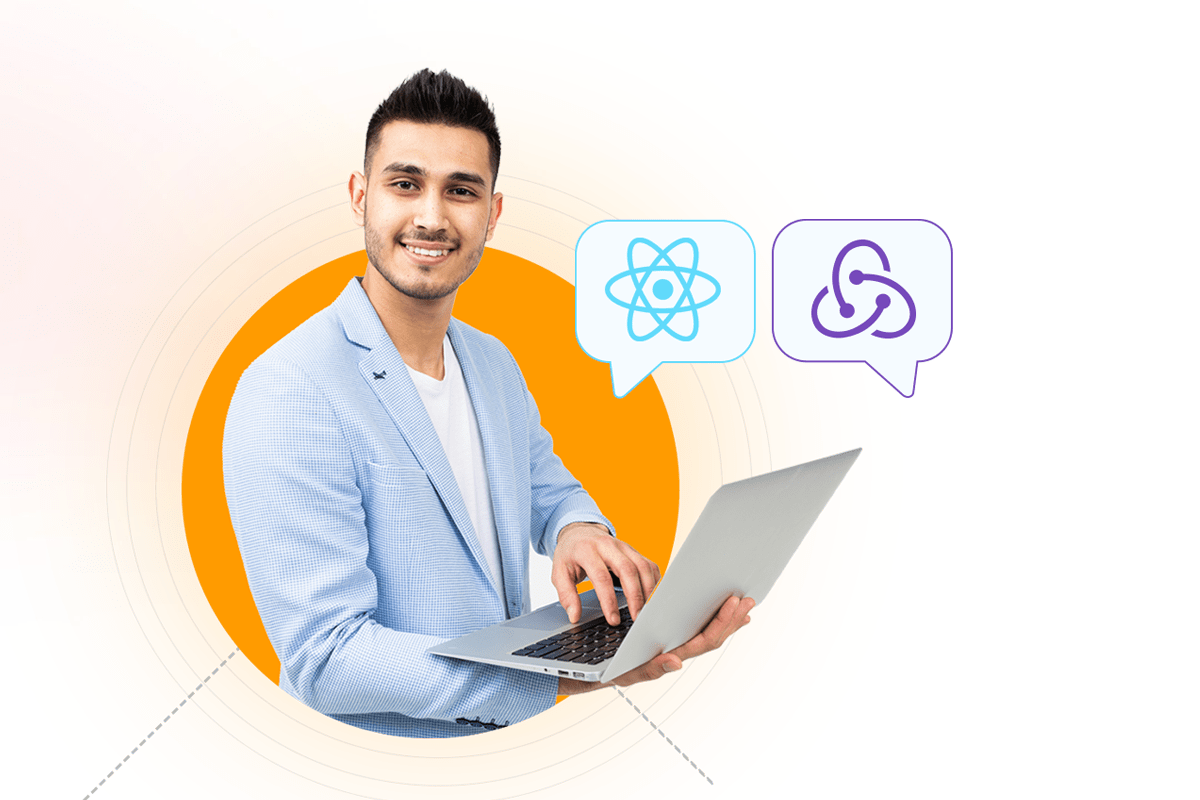
In Today’s world when we think of creating new web applications, there are a number of library/ framework that comes into our head that can be used to create a web application in an easy way.
It’s true because, In today’s world, we have numbers of libraries/frameworks like ReactJS, Angular, and Vue using which you can easily create new web applications really quickly.
But have you thought about how data are managed inside any application? In a large application, we have a number of components that share data with each other, making the network-like structure and making your application very hard to understand and debug.
And if you have to extend your application or add new features. It’s a headache for many developers. So let’s discuss how we can manage data in an easy way.
Before jumping into how we can manage data inside your application let’s try to understand what are possible ways to pass data between components.
In the first case, all the components are sharing data with each other which is very hard to understand the flow of data between components. And all the component is dependent on each other to share data. So if you will make changes to any component you have to be very careful because it will affect others as well
In the second case, there is one store that holds all the data, and whatever component needs data that will look inside the store. And it’s totally independent of another component. So making changes to any component will not affect the other one.
After comparing both the way you must be curious to know more about Redux. Like what is redux and how it manages data for applications.
As we know Redux is a library that we have to install in our application which will take care of all the state of our application. You must be thinking of localStorage, session storage, or a cookie that can also be used to store data in a single place and a component can get data from there then why use Redux?
So Redux and localStorage have different use cases. Redux you will use to manage your application state across multiple components. Local Storage you will use to persist properties in the browser for later usage. The actual problem is that any changes in your local Storage or session storage won’t reflect on your application.
So let’s see what redux is and how it manages the state for our application.
Redux is a Javascript library that does state management for your application. Same as like context does. Context use provider and consumer to provide data among component which create a portal to skip passing parameter through every component. With Redux you can take the state management out of your library entirely and move it to a separate store. It is quite similar to a Chartered Accountant who is not part of your organization but handling the finance-related stuff for your organization
Components of Redux
Redux is divided into 3 components which are Action, Reducer, and Store.
Store -> Store is a single place where all the data is stored so whatever component needs data it will directly get from the store. That’s why the store is a single source of truth for the application.
Action -> an Action is an object that includes 2 properties Type and Data. Here type tells what type of operation you want to perform and what data is like while performing that operation if you need some data.
Reducer -> Reducer is a function that is responsible to make changes in the store. Depending on the action type reducer perform the operation to update the store state.
So whenever any action will dispatch reducer will run and it will make changes in the store if the store will be updated it will re-render the component in which you are getting data from the store.
This is the workflow of Redux. Let’s try to understand this workflow with code examples in React application.
Let’s try to understand the redux workflow with a code example that will create a bug-tracking application in which we can add bugs, and remove the bug.
In your project folder install two libraries => reduxjs/toolkit and react-redux.
To do that run this command in your terminal => “npm install @reduxjs/toolkit react-redux”
Create a new file store.js and put in it ->
Here we pass the reducer in store So whatever reducer will return will be the initial state of the store.
To create reducer and actions create new folder slices and inside that create a file bugSlice.js
Reducer is a function that will take two argument states and action and depending on the action type it will return a different object and that returned object will be the final state of store.
Now to provide the value of store to all of the components of your react application go to index.js and put in it.
Here I have wrapped the App component with Provider and provided the store.
So that any component inside of your application can communicate with the store.
To get data from the store add a few lines as I have added on PrintBug.js
In the console, you can see all the bugs from the store.
And to add a new bug in your store you have to dispatch the addBug action
Whenever dispatch will run it will call reducer with the object inside it.
And it will add bug1 to your bugs array.
To remove the bug just dispatch the action removeBug. And payload with an id of the bug you want to remove.
Like dispatch(removeBug(1));
It will remove the bug whose id will be 1.
Conclusion: Redux provides a structured approach for managing application states, simplifying the process of understanding and debugging complex state interactions. By establishing a single source of truth and enforcing a unidirectional data flow, Redux facilitates the creation of maintainable and scalable JavaScript applications.