How to Develop a Microservices-Based Architecture with Python – Introduction
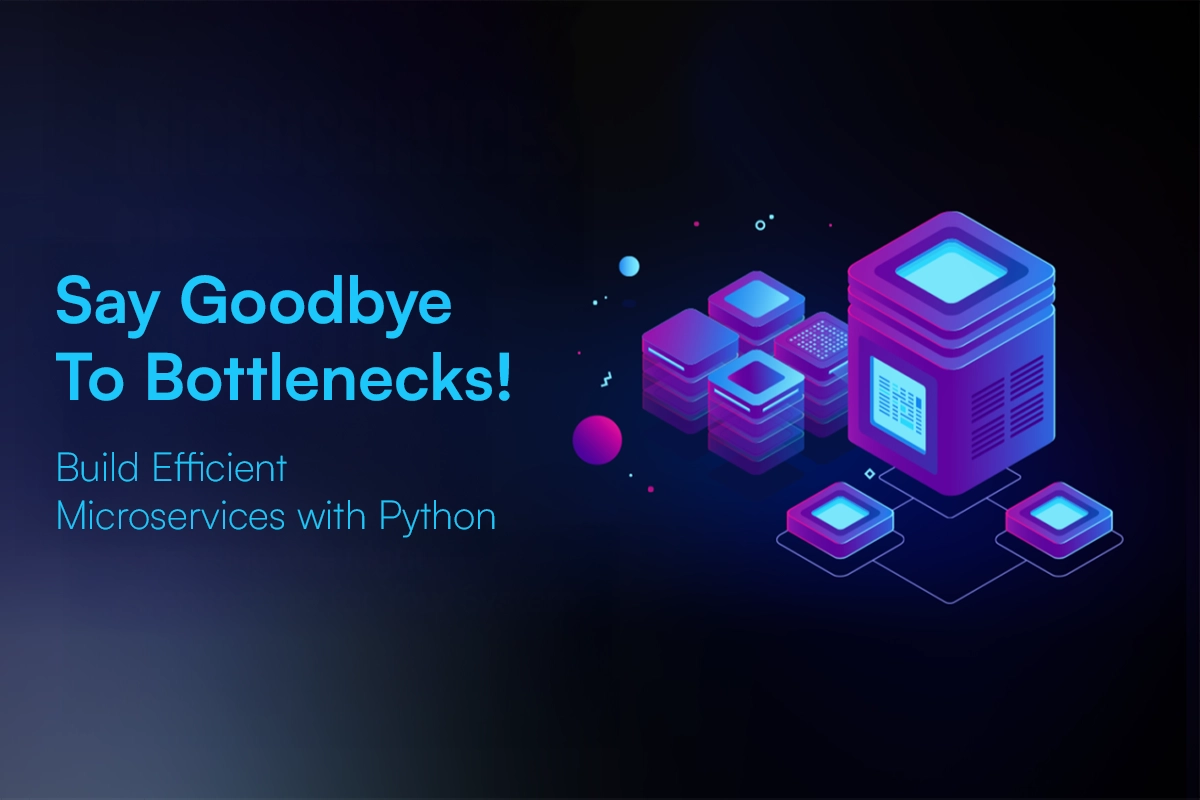
1. Introduction: Why Microservices and Why Python?
In the early days of software development, most applications were built using a monolithic architecture, where all components—backend logic, database, and frontend—were tightly coupled into a single codebase. While this setup works well for small projects, it often leads to scalability and maintenance challenges as the application grows.
Remember when all software lived in one massive package? That’s the monolithic approach – imagine trying to renovate an entire house at once! While this worked for smaller projects, it’s like trying to squeeze an elephant through a doorway when your application grows.
Microservices offer a modern alternative by breaking down an application into smaller, independent services that handle specific functions, such as user authentication, payment processing, or order management. Each service operates separately but communicates with others to form a complete system.
Benefits of Microservices:
- Scalability – Each service can scale independently based on demand. For example, if a payment service experiences heavy traffic, only that service needs to be scaled instead of the entire application.
- Flexibility – Different services can use different technologies and databases. This allows teams to choose the best tools for each function.
- Fault Isolation – A failure in one service does not bring down the entire system. If a messaging service crashes, the rest of the application continues running.
Why Python for Microservices?
Python is one of the great choices for developing microservices due to its simplicity, rapid development capabilities, and strong ecosystem. Which allows developers to build and deploy scalable services efficiently while ensuring seamless communication between different components of a microservices architecture.
- Rich Ecosystem – Python offers powerful frameworks like FastAPI, Flask, and Django, which make it easy to build lightweight microservices.
- Rapid Development – Python’s clean syntax speeds up coding, making it ideal for fast iterations.
- Easy Integration – Python supports REST, gRPC, and messaging queues like Kafka and RabbitMQ, making service-to-service communication seamless.
Because of these advantages, many modern companies, including Netflix, Uber, and Instagram, use Python for their microservices architecture.
2. Core Principles of Microservices
Now that we understand why microservices and Python are a great combination, let’s move to the next step—the core principles that shape a microservices-based architecture. Following these principles ensures that your system remains scalable, flexible, and maintainable as it grows.
Here are some of the most important concepts:
Microservices Should Be Independent and Stateless
Unlike monolithic applications, where all components are tightly coupled, microservices should be independent and stateless whenever possible. This means:
- Each service has its own codebase, database, and dependencies.
- Services can scale independently without affecting others.
- A failure in one service does not impact the entire system.
Stateless microservices process each request independently, improving scalability and fault tolerance. However, if stateful services are necessary (e.g., shopping carts or session tracking), they should use external storage solutions like Redis or databases instead of relying on in-memory storage.
Choosing the Right Communication Method
Since microservices must communicate efficiently, selecting the right method is crucial:
- REST APIs – Best for simple, synchronous request-response communication (e.g., public-facing APIs).
- gRPC – Ideal for high-performance, low-latency interactions, especially for inter-service communication.
- Message Queues (Kafka, RabbitMQ) – Used for asynchronous, event-driven workflows, reducing dependencies between services.
By following these principles, microservices remain scalable, fault-tolerant, and efficient, ensuring a well-structured and reliable system.
3. Setting Up Your Python Microservices Project
Now that we’ve covered the core principles of microservices, let’s move to the next step—setting up a Python microservices project. A well-structured setup ensures better scalability, maintainability, and deployment flexibility
Choosing the Right Framework for Microservices
Python offers multiple frameworks for building microservices, each suited to different needs. Here’s a quick comparison to help you choose the best one for your project:
Framework | Best For | Pros | Cons |
FastAPI | High-performance APIs | Async support, built-in validation, automatic docs | Newer ecosystem |
Flask | Flask Lightweight services | Minimal, flexible, large community | Requires manual configuration for scalability |
Django | Full-fledged web apps | Security, ORM, batteries-included | Heavy for simple microservices |
Tornado | Real-time applications | WebSockets, non-blocking I/O | Smaller community, complex setup |
Choosing the right framework is crucial for building efficient microservices. FastAPI is ideal for high-performance, modern APIs, while Flask works well for lightweight services. If your microservice requires built-in authentication, ORM, or an admin panel, Django can be a great choice. For real-time applications, Tornado is worth considering.
Structuring the Microservices Repository
A well-organized repository structure helps teams collaborate efficiently and manage services independently.
Here’s a typical monorepo structure (keeping all microservices in one repository):
Alternatively, in a polyrepo setup (each microservice has its own repo), you’d manage them separately and connect them using an API gateway.
Best Practices:
- Keep microservices independent, with their own requirements.txt and dependencies.
- Use a common module (/common/) for shared utilities like logging and email service.
- Maintain separate Dockerfiles for each microservice.
- Use an API Gateway to route traffic efficiently.
Using Docker for Containerization
Docker makes microservice deployment easier by running each service in its own container with all required dependencies. It ensures consistency across different environments, making deployments more portable, scalable, and reliable. With tools like Docker Compose and Kubernetes, managing and orchestrating microservices becomes seamless. By containerizing your services, you can improve resource efficiency, speed up deployments, and enhance scalability, leading to a more robust and efficient architecture.
Example: Dockerfile for a FastAPI Microservice
Running a Microservice with Docker
docker build -t auth_service .
docker run -p 8000:8000 auth_service
Meet CloudLaunchPad: Your Gateway to Effortless Infrastructure Automation for Scalable Microservices!
Setting up infrastructure for microservices can be complex, requiring network configurations, deployments, and monitoring setups.
CloudLaunchpad can help in automating infrastructure setup for microservices-based projects by providing streamlined deployment, scaling, and security configurations. Instead of manually provisioning servers, containers, and databases, Cloud Launchpad simplifies the process, making deployments faster and more reliable.