A Deep Dive into Mastering API Development
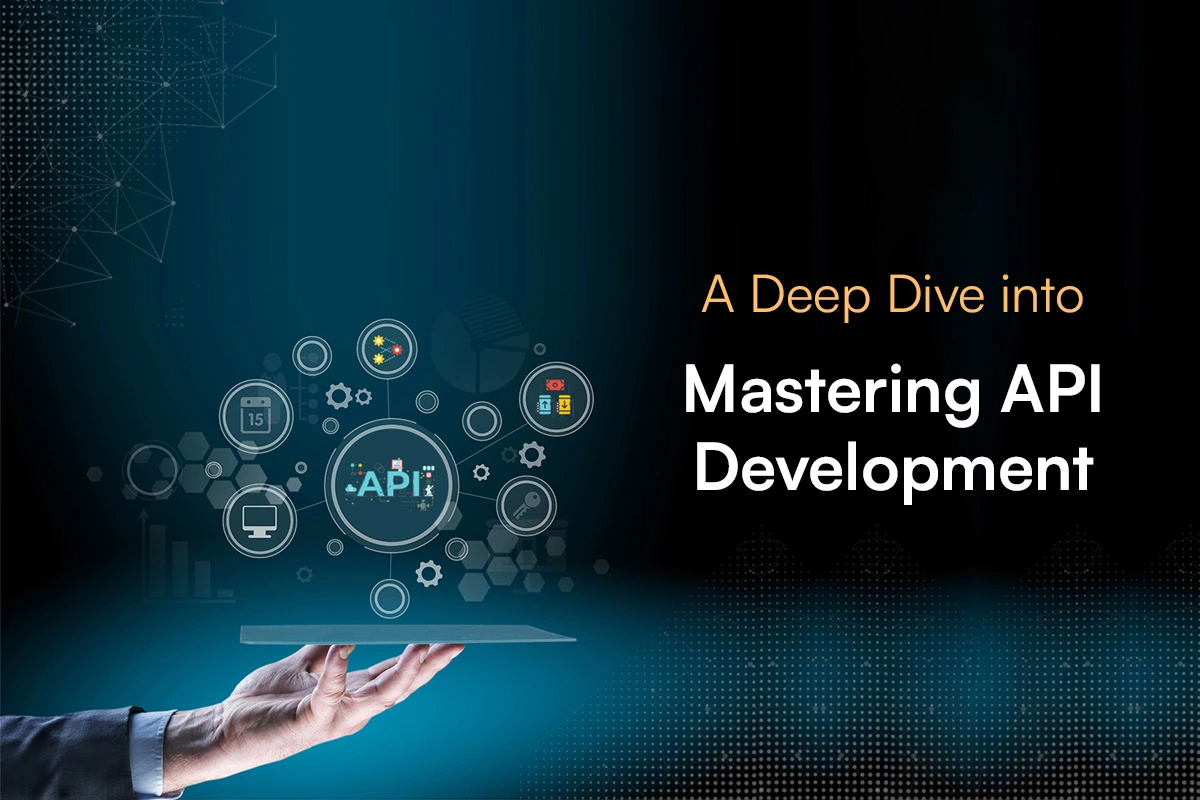
In our First blog on API development, we started our journey into the fundamentals of APIs, explored various API types, and discussed essential principles. If you have not read it yet, you can find it HERE. In this second part of our guide, we will continue our journey through the world of API development and management, focusing on important aspects like request and response handling, authentication, API documentation, and testing.
6. Request and Response Handling
a. How to structure API requests
Effective request and response handling are essential for designing APIs that function seamlessly. Here we explore the key components of this process:
Headers
HTTP headers provide additional information about the request and are essential for various purposes, including authentication and content metadata. Common headers include:
- Content-Type: Specifies the format of the request body data (e.g., JSON or XML).
- Authorization: Used for authentication, it includes credentials or tokens to identify the requester.
- Accept: Specifies the preferred response format (e.g., JSON, XML, or HTML).
Although there are many more headers available, while developing APIs, these three are the ones used most frequently.
Query Parameters
Query parameters allow the client to customize the request by setting specific properties in the URL. These properties, more commonly known as parameters, are often used for filtering, sorting, pagination etc.
For example, in a RESTful API for movies, you might have query parameters like ?genre=horror or ?page=2&pageSize=10 to retrieve horror movies or set the page to 2 and get only 10 results. There are numerous other things that it can be used for, like for sending search terms, setting API keys for public APIs etc. Keep in mind, they are used with GET requests only in most if not all cases.
Although as convenient as it may seem, using and relying on parameters are not always a great idea. If the data you’re sending has any kind of sensitive information, it is never recommended to use query parameters. It should mostly be kept as a way to transmit metadata and non-sensitive information that relates to the data you’re requesting. Also, very long parameters should be ideally avoided and names should be easily understandable by the end user.
Most API frameworks in any programming language will give you easy access to these query parameters whenever you are parsing a request as it is an extremely common pattern.
Request Bodies
In many cases, you need to send data to the server. This is common in POST and PUT requests when creating or updating a piece of data in the server. The request body typically contains data in JSON or XML format. Same as query parameters, it is often easily available or automatically parsed by any framework you might be using. For example, in a movies JSON API for creating a new movie, the request body could look like this:
Code:
b. Handling different types of responses
When you make a request to the server, the API can have a multitude of responses depending on what you requested, status of the server, request structure etc. It is crucial to send consistent and meaningful data back to the client and handle edge cases properly.
Successful Responses
A successful response typically includes a 2xx status code (e.g. 200 OK) and the requested data. Clients should parse and use this data as needed. For example, if you request a list of movies, the response might look like this:
As you can see, all the entries returned by the API have the same structure and are often considered a good practice unless there is an exception. The format and structure can vary greatly depending on what the client requested.
Error Responses
Error responses come with status codes in the 4xx or 5xx range. It is returned when something goes wrong with the request, either due to server issue, authentication issue or something else. It is often returned with status codes between 4xx and 5xx range. Effective error handling is essential to guide developers when issues arise, as without proper error messages, it would be impossible for the consumer of the API to know why the issue is arising. For example, a 404 response indicates that the requested resource was not found while a 401 means that it might have been found but you are not authorized to get it.
Here is an example of error message:
As you may notice, it is common to send the generic error type, the status code, and the detailed message of what exactly happened. The message part is often more important than what people give it credit for, as good, detailed messages can reduce hours of development time if an error arises for the API consumer.
Pagination
When dealing with large datasets, which is quite common in real world APIs, we often implement pagination. Pagination typically includes metadata in the response to indicate the current page, the total number of pages, the number of entries in the current page and total entries all together.
This is sent alongside the data on paginated requests, which as mentioned earlier, are sent through query parameters. For example, a query request like ?page=2&pageSize=10 might result in the data send back, to look like this:
7. Authentication and Security
a. Importance of API authentication
API authentication is a fundamental aspect of API security that safeguards data and prevents unauthorized access. It acts as a barrier, ensuring that only the entities that should have access to the data, get access to the data.
As APIs often carry sensitive data over the internet, ensuring that authentication is setup properly is of paramount importance to prevent malicious attacks and unauthorized usage.
b. Overview of authentication methods
There are many standard ways to secure an API in the industry that we use today and they vary depending on your use case and needs of your API.
Here are some of them:
1. API Keys:
API keys are simple tokens (often long strings of characters) that clients use to authenticate with an API. They are typically sent as a header or query parameter in API requests.
API keys are suitable for scenarios where simplicity and ease of use are essential, such as accessing public APIs or internal APIs with limited security requirements. Anything with high security or carrying sensitive information, should not rely solely on this method.
2. OAuth:
OAuth is an authorization framework that allows third-party applications to access resources on behalf of a user without exposing the user’s credentials. OAuth 2.0 is the latest and most widely used version.
OAuth is ideal for securing APIs that require user-specific data, such as social media logins, access to user profiles, or authorization for third-party apps.
It is a robust and standardized way to handle authentication and authorization and allows features such as limited access to users, scoped access to data and is also extensible.
3. JWT (JSON Web Tokens):
JWT is a compact, self-contained token format for securely transmitting information between parties as a JSON object. JWTs can be used for authentication and authorization.
JWTs are stateless and relatively simple, making them suitable for custom needs with distributed usage. They can carry claims (e.g., user ID, roles) and are signed, ensuring data integrity. They are often chosen when you need a lightweight, token-based authentication mechanism with scalability.
The choice of authentication method depends on the specific requirements of your application, including security, user experience, and integration complexity. OAuth and JWT are often favored for securing user-specific data, while API keys can be suitable for simpler or internal use cases.
c. Best practices for securing APIs
Securing APIs is crucial for protecting sensitive data, ensuring the integrity of your systems, and preventing unauthorized access or attacks. Here are some best practices for securing APIs:
- Authentication and Authorization: Employ robust authentication techniques to guarantee that only legitimate users or authorized applications can interact with your API. As discussed earlier in this article, each mechanism has its own pros and cons, and should be considered before implementation.
- Use HTTPS: Always use HTTPS for API communication to encrypt data in transit and prevent man-in-the-middle attacks.
- Rate Limiting: Implement rate limiting in some form to prevent abuse or overuse of your API resources. This also helps mitigate DoS (Denial of Service) attacks.
- Input Validation and Sanitization: Validate and sanitize all input data to prevent injection attacks like SQL injection, Cross-Site Scripting (XSS), and other vulnerabilities.
- Security Headers: Implement security headers like Content Security Policy (CSP), Cross-Origin Resource Sharing (CORS) policies, and X-Content-Type-Options to mitigate various web security risks.
Other than these, there are other things to keep in consideration such as proper monitoring and logging of endpoints, API versioning and deprecation and internal connections. Also if your API is using any third party APIs, always ensure you are securely connecting to them and never expose or store your API keys in public repos or code.
8. API Documentation
a. The significance of clear and comprehensive API documentation
Clear and comprehensive API documentation is a game-changer for developer adoption and user satisfaction for several important reasons:
- Ease of Integration: Well-documented APIs provide developers with clear and concise instructions on how to integrate and use the API in their applications. This reduces the learning curve and speeds up the development process, making it easier for developers to adopt and work with the API.
- Reduced Support Burden: When API documentation is thorough and understandable, developers are less likely to encounter issues or have questions during the integration process. This reduces the need for developers to seek help from your backend team, saving both time and resources.
- Standardization: Comprehensive documentation often includes best practices, coding guidelines, and usage patterns. This creates standardized coding practices resulting in more consistent and reliable implementations.
- Security and Compliance: Documentation can include information on security best practices and compliance requirements, helping developers ensure that their implementations are secure and meet industry standards.
- Updates and Versioning: API versioning can often change throughout the development lifecycle, and having proper versioning for it is important to create distinct boundaries for different API versions.
Basically, clear and comprehensive API documentation significantly contributes to developer experice by streamlining integration and reducing help seeking and greatly helps in reducing the friction between backend and frontend teams, which is very important to the success of your platform.
b. Tools and formats for generating API documentation
There are various tools available in market that help speed up the process, but here are two of the most used ones:
1. OpenAPI: OpenAPI is a widely adopted standard for describing RESTful APIs. It allows you to define endpoints, request/response formats, authentication methods, and more in a machine-readable format. Tools like Swagger UI can generate interactive API documentation with proper UI that can be easily hosted, from OpenAPI specifications. It might lack in show and glamor, but it makes up for it in stability, extensibility, and resources available for it on the internet.
2. Postman: Postman is a tool for API testing in general, but with the introduction of API collections, it has also become a key player in the API documentation market. It provides tools like API Designer and provides truly clear and concise ways to define each endpoint, their query parameters, request body, and headers. What sets it apart is that it is also extremely easy to make and test requests as it was initially a testing tool. It also has a very robust set of features like real time collaboration, global variables etc.
9. Testing APIs
a. Strategies for testing API endpoints
Testing API endpoints is crucial to ensure the reliability and functionality of your APIs. Here are some strategies for testing API endpoints:
- Manual Testing: Manual testing involves manually sending requests to API endpoints using tools like Postman or curl. It is a good way to get started and understand how the API works. Manual testing can help identify basic issues and validate functionality.
- Unit Testing: Unit testing focuses on testing individual units or components of an API in isolation, such as specific endpoints or functions. This type of testing ensures that each component works as expected. It often involves writing test cases using testing frameworks like JUnit.
- Performance Testing: Performance testing assesses how the API performs under different loads and conditions. It includes load testing, stress testing, and scalability testing.
- Security Testing: Security testing checks for vulnerabilities and threats in the API, such as SQL injection, cross-site scripting (XSS), and authentication vulnerabilities. Tools like OWASP ZAP and Nessus can help identify security issues.
b. Overview of unit testing, integration testing, and mocking
- Unit Testing: Unit testing focuses on testing individual units or components of an API in isolation. In API testing, this might involve testing specific endpoints or functions. Unit tests ensure that these individual units behave as expected and help identify bugs early in development. Mocking frameworks are often used to simulate dependencies and isolate the unit being tested.
- Integration Testing: Integration testing checks the interactions between different components or endpoints within an API. In API testing, this involves testing how different parts of the API work together. Integration tests verify that data flows correctly between endpoints and that the API components communicate as intended.
- Mocking: Mocking is a technique used in unit and integration testing to simulate dependencies that an API relies on but are not under test. Instead of using real external services or components, mock objects or functions are created to mimic their behavior. This allows testers to isolate the unit or integration being tested, making tests more predictable and controllable.
c. Introduction to API testing tools
- Postman: Postman is a widely used tool for manual and automated API testing. It provides a user-friendly interface to send requests, manage test cases, and generate test scripts.
- Swagger (OpenAPI): Swagger is an API specification framework that allows you to define, document, and test APIs. Tools like Swagger UI and Swagger Inspector can help you test your APIs based on the OpenAPI specification.
- SoapUI: SoapUI is a tool for testing SOAP and REST APIs. It offers features for functional testing, load testing, and security testing of APIs.
- Jest: Jest is a JavaScript testing framework often used for testing APIs in Node.js applications. It includes built-in mocking capabilities for isolating units during testing.
Conclusion
In this article, we have dived deeper into the world of API development, covering topics like request and response handling, authentication and security and API documentation. By mastering these aspects, you will be better equipped to create robust, secure, and well-documented APIs that meet the needs of both developers and users. Stay tuned for the next part of our guide, where we will explore advanced topics in API development and management.