Decoding Microservices: Architecture and Design Principles
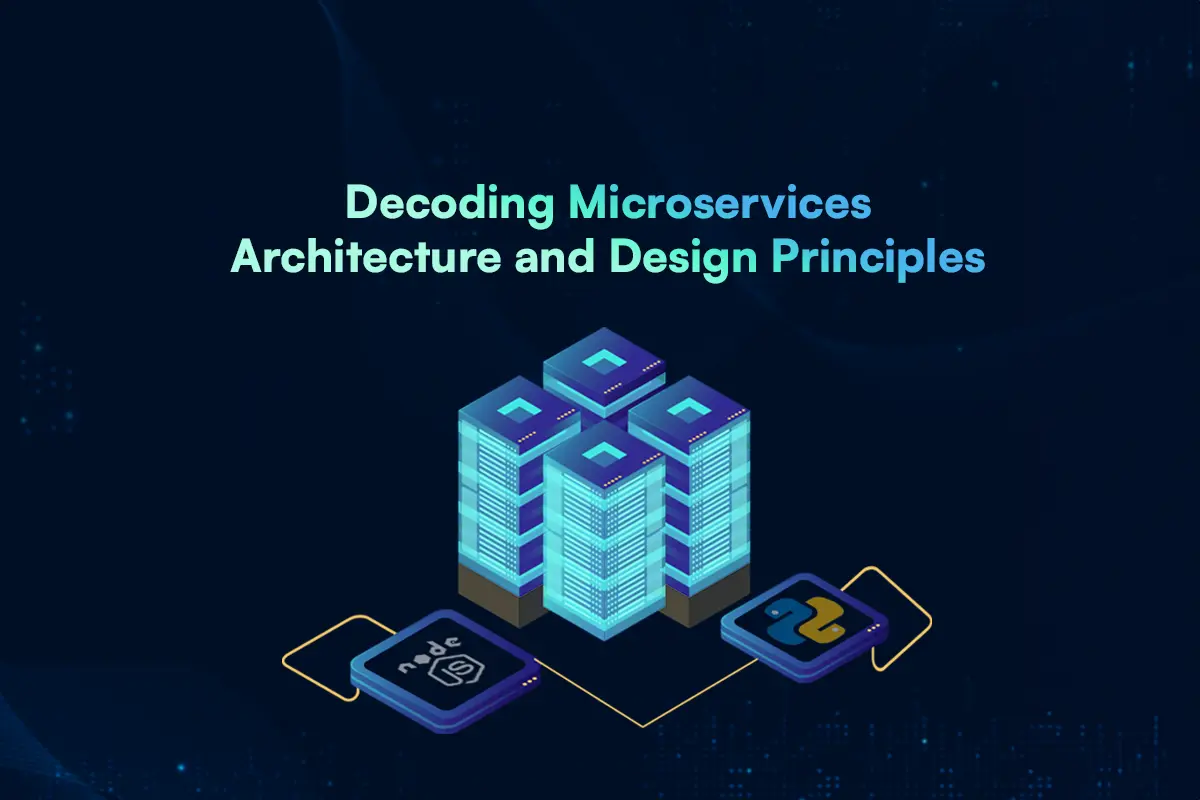
Decoding Microservices
Alright, let’s kick things off! Since this article is packed with details, I’ll save you from information overload by splitting it into two parts. In this first part, we’ll dive into the architectural nitty-gritty of microservices and take a bird’s-eye view of the system design. In the next part, we’ll get our hands dirty by writing some code and bringing those designs to life. Hopefully, you’ll find this useful—and if you do, please let me know so I can brag about it later!
Now, let’s answer the burning question: What exactly are microservices?
if we go by a textbook definition it says , Microservices are
But what does it actually mean ? so, to answer that let’s shade some light on traditional monolith architecture first
Monolith Architecture
- This is a simple representation of a monolith architecture of a very low budget e-commerce app. so it might have a Authentication feature where users can be authenticated and may be authorized with the help of JWT token for example.
- then it might have a user feature handling all registered users ,a product feature will have a catalog of products such as overly priced headphones, towels and what not.
- The order feature will represent a user who ordered it relating to the products and last but not least it will have a payment feature to pay for these orders.
- Also it’s worth mentioning that it uses a single database structure, where all these features are dumped under the tables.
- For simplicity’s sake we are keeping it limited, and I would like you to notice that all the features are Jam packed like a traveling bag under one single app.
Disadvantages of Monolithic architecture
Although I can write about its disadvantages for at least a week, let’s mention only major disadvantages here.
Hard to Manage Codebase
- Code Organization and Modularization:
- Imagine a giant bowl of spaghetti, each noodle representing a line of code. Now, picture trying to extract just one noodle without disturbing the rest. That’s your codebase.
- Lack of Clear Boundaries:
- There are chances of code getting so messed up that over time it will resemble an over working dev living in a studio apartment where his bed, kitchen, and office are all in one room. Good luck finding a charger in that mess.
- Inconsistent Coding Styles:
- The codebase will have been exposed to so many developers in and out. it will look like a patchwork quilt made by interns, junior devs, senior devs, and that one guy who insists on using tabs over spaces. Each patch tells its own confusing story.
Not Scalable
- Tightly Coupled Components:
- Scaling this monolith is like trying to change a car’s tire while it’s speeding down the highway. Everything is so intertwined, you’d need a miracle (or a pit crew) to pull it off.
- Single Point of Failure:
- There is a clear chance of a single point of failure like It’s like having a single power switch for your entire house. Flip it off, and suddenly you’re in the dark, with no way to find the bathroom at night.
- Resource Contention:
- It also has high resource contention due to a single shared database. All features sharing a single database is like a family of ten sharing one bathroom. When everyone needs it at the same time, chaos ensues.
- Deployment Headaches:
- Introducing updates and deployment is also not enjoyable.
- Updating a monolithic app is like trying to repair a leaking tap in the middle of a busy wedding feast. One small mistake, and you have a mess on your hands while everyone’s watching.
Maintenance and Flexibility
- Limited Reusability:
- Your code is so intertwined that reusing parts of it is like trying to extract a single Lego piece from a glued-together sculpture. It’s more trouble than it’s worth.
- Tech Debt:
- Over time, the monolith accumulates technical debt faster than a teenager with a credit card. And just like financial debt, it’s a pain to pay off.
Development Speed
- Slower Development Cycles:
- development speed is also affected due to such architecture.
- Onboarding New Developers:
- We all have been in these situations where we joined a new company and were introduced to their humongous codebase which may be they themselves hardly understand.
- New developers face a learning curve steeper than Mount Everest. By the time they understand the codebase, they might already be looking for their next job.
I think these are sufficient to make the point here.so What is the alternative ? The Microservices !
Microservice Architecture
- so if we were to develop that same e-commerce app but in a Microservice architecture , this is what it will look like. Each feature is a service and also they all are independent and they all have their individual database. They all connected to a single API gateway for communication. This is in a nutshell a basic representation of Microservice architecture.
Quick Comparison
Though comparing them may not be entirely fair , like a kid being compared to the class nerd by their parents but it does give some perspective on their operational differences.
I’ve added a new feature of notifications just to make it look more appealing .
Each microservice can be containerized using Docker and act as a single deployable unit, connected via exposed APIs through ports, and work independently.
- In a monolithic app, everything is developed within a single framework. In contrast, microservices offer the advantage of using several different languages, allowing developers to choose the best tool for each task.
- This flexibility ensures that no developer has to sit on the bench or work on in-house projects just because their preferred language or framework isn’t being used. Plus, with microservices, it’s easier to scale up or down a particular service based on demand and business needs. This agility can save costs you’d otherwise spend on cloud hosting providers.
So now to the question, how to develop a production grade microservice using node.js or python ?
let’s do it by developing an imaginary food delivery app “MealZip ”.
here is short Business requirement of “MealZip”
- Our app will connect users with local restaurants, enabling smooth ordering and efficient delivery.
- It will feature user registration, profile management, and secure payments.
- Restaurants will manage their listings and menus, while users can track orders and receive real-time notifications.
- The app will handle delivery logistics, manage promotions, and include a review system.
- Built with a microservices architecture and Docker for scalability and independent deployment, our app aims to provide a seamless, reliable experience as it grows. here are characters related to Our App
Possible Services
- User Service: Handles user authentication, profile management, and user preferences.
- Restaurant Service: Manages restaurant listings, details, and availability.
- Menu Service: Manages menu items, pricing, and availability for each restaurant.
- Order Service: Handles order creation, tracking, and history.
- Payment Service: Manages payment processing, transaction history, and refunds.
- Notification Service: Sends notifications to users and restaurants about order status, promotions, and updates.
- Delivery Service: Manages delivery logistics, driver assignments, and tracking.
- Review Service: Handles user reviews and ratings for restaurants and delivery experiences.
Tech stack
Microservices
- Express: For creating RESTful APIs with Node.js microservices.
Databases
- MongoDB: For storing flexible and scalable data (used by some microservices).
- PostgreSQL: For relational data management (if needed).
Caching
- Redis: For caching frequently accessed data to improve performance.
Messaging
- Kafka: For real-time data streaming and inter-service communication.
Containerization
- Docker: For containerizing microservices to ensure consistent development and deployment environments.
Orchestration
- Kubernetes: For managing and orchestrating Docker containers, including scaling and deployment.
Payment Processing
- Stripe/PayPal: For handling payment transactions.
Notifications
- Twilio/SendGrid: For sending SMS and email notifications.
Infrastructure and DevOps
- AWS/GCP/Azure: For cloud hosting and infrastructure services.
- Git: For version control.
- Jenkins/GitHub Actions: For CI/CD pipelines.
Logical Architecture of the App
The Great Food Delivery Adventure in Microservice Realm
Chapter 1: The Hungry Hero
Meet John, our modern-day food lover, scrolling through the app like it’s Tinder for food. After a solid five minutes of indecision, John finally taps “Order” on a gourmet burger. Little does John know, this one click kicks off a digital frenzy.
Chapter 2: The Order Frenzy
- Enter Order Service, the unsung hero behind the scenes.
- Order Service: “Oh Great, another order. Let’s get this show on the road!”
- It logs the order and sends it to the Kafka Queue, the ultimate middleman, making sure everyone in the Microservice realm hears the news.
- Meanwhile, in the heart of the kitchen, Restaurant Service is updating the menu.
- Restaurant Service: “Better keep things fresh, or John might think we’re out of ideas.”
- It sends the menu update back through the Kafka Queue. Everyone in the land now knows the menu has been spruced up.
Chapter 3: The Payment Saga
- Now it’s time for Payment Service to step in, flexing its transactional muscles.
- Payment Service: “Alright, John. Time to part with your precious cash.”
- It engages in a battle of bytes with the Payment Gateways, ensuring the money flows smoothly into the restaurant’s coffers. Successful, it updates its records and pings the Notification Service.
- Notification Service: “Hey John, your payment is done. Fingers crossed it was worth it!”
Chapter 4: The Delivery Dash
- With the payment sorted, it’s now Delivery Service’s turn to shine.
- Delivery Service: “Alright, time to earn my keep! Let’s get this food on the road.”
- It dispatches the nearest rider and tracks every movement with the enthusiasm of a fitness app. The Delivery Personnel Interface updates continuously, making sure the rider stays on track.
- Notification Service: “Hey John, your food’s en route. Sit tight and stop checking the app every 30 seconds.”
- Meanwhile, the delivery guy, armed with a questionable sense of direction and a knack for getting lost, begins his epic journey. After roaming all around the neighborhood and calling John multiple times for directions, he finally arrives with the precious cargo.
- Delivery Guy: “Here’s your food. Hope it was worth the adventure!”
Chapter 5: The Review Rampage
- After devouring the delicious burger, John feels compelled to share the experience.
- User Interface: “Got feedback? We’re all ears!”
- Review Service catches wind of this and logs the review with gleeful efficiency.
- Review Service: “Nice! Another review in the bag. Let’s broadcast this to everyone!”
- The Restaurant Service gets the notification and hopes for the best.
- Restaurant Service: “Please be a good review. Please give me a good review…”
- Epilogue: The Notification Overload
- Through it all, the Notification Service buzzes relentlessly, keeping everyone informed.
- Notification Service: “Updates! For everyone! John, your food’s on its way. Restaurant, you’ve got a new review. Delivery guy, keep moving!”
And so, the great food delivery adventure concludes. John’s can finally binge watch some Netflix series and enjoy the food. Thanks for helping John with his appetite we will cover Deployment strategy and coding details in next.
Once again Thanks for tuning in,
Catch you in the next sprint!