Decoding Microservices: Implementation in Node.js and Python
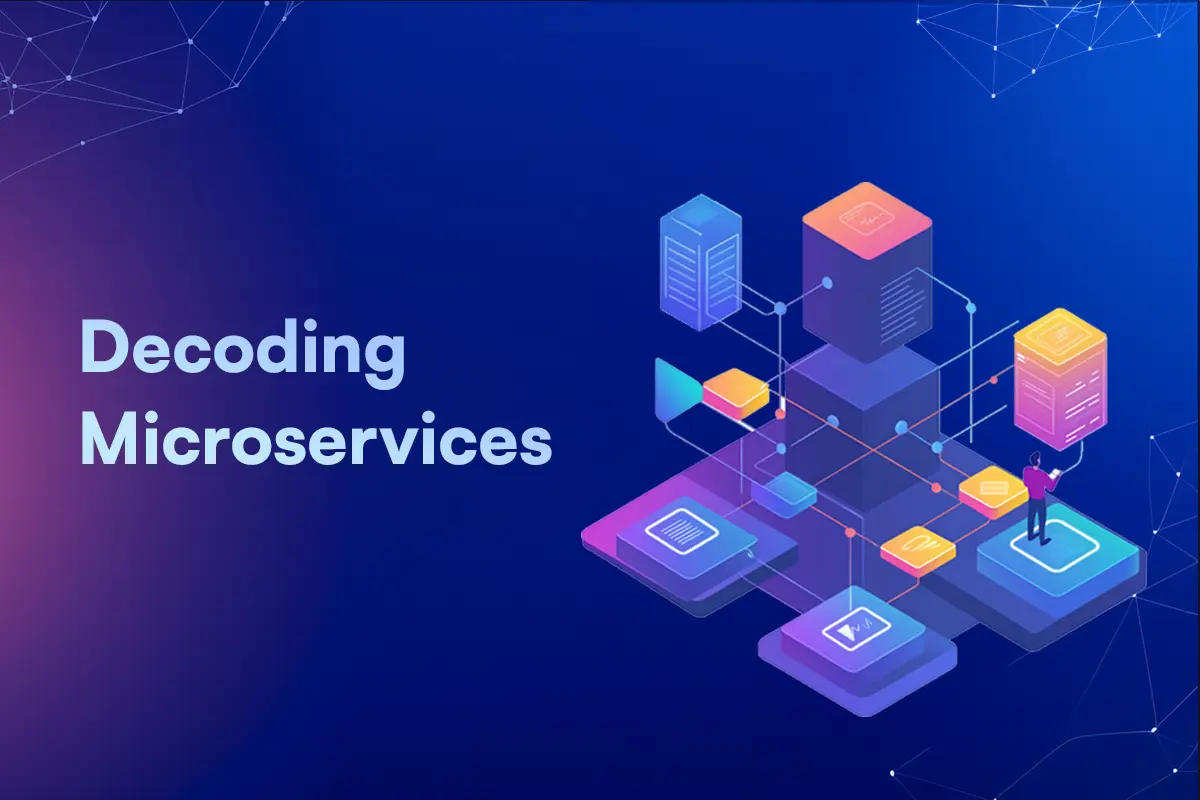
Alright, time to roll up our sleeves! First part was packed with details, In this part, we’ll take all that architectural theory and turn it into actual code. No more just talking about how cool microservices are—it’s time to show it. So, grab your favorite coding snack, because we’re diving in!
By the end of this, you’ll not only understand how microservices work, but you’ll also have some insight for having built one yourself. Let’s get started!
Deployment strategy
This is a simple representation of a microservices architecture deployed using Azure Cloud Services, Kubernetes, and Nginx, catering to a moderately scaled application.
In this setup, we have distinct components serving various parts of our application, ensuring they are modular and independently deployable:
- Frontend:
- React Application: Our user interface is built using React, encapsulated in a Docker container for ease of deployment.
- Azure Cloud Service: This container is deployed to an Azure cloud service, ensuring high availability and managed hosting.
- Kubernetes: The frontend is further deployed to a Kubernetes cluster, allowing for scaling, rolling updates, and orchestration of multiple instances of our React app.
- Backend:
- Node.js Application: The server-side logic is implemented using Node.js, also containerized using Docker.
- Azure Cloud Service: Similar to the frontend, the backend container is hosted on Azure.
- Kubernetes: Deployed to a Kubernetes cluster, our backend benefits from Kubernetes’ ability to manage container lifecycles, auto-scale, and recover from failures.
- Database:
- MongoDB: Our primary database is MongoDB, hosted in a Docker container. It provides a flexible schema and is ideal for handling the various types of data our application generates.
- Caching:
- Redis: For caching, we use Redis, also containerized. It helps speed up data retrieval and reduce the load on our primary database by storing frequently accessed data.
- Ingress and Load Balancer:
- Ingress Nginx: Acting as the entry point for our application, Ingress Nginx routes incoming traffic to the appropriate service (frontend or backend). It provides a single point of entry, simplifying the URL structure for clients and enabling SSL termination.
- Load Balancer: This component evenly distributes incoming requests across multiple instances of our services (frontend and backend). By doing so, it ensures no single instance is overwhelmed, thereby improving performance and reliability.
When a client interacts with our application, here’s what happens:
- The client sends a request, which first hits the Ingress Nginx.
- Ingress Nginx examines the request and routes it to the appropriate service (e.g., frontend for UI, backend for data processing).
- If the request requires data, the backend may fetch it from MongoDB or Redis.
- The Load Balancer ensures that each request is distributed to the least loaded instance of the requested service, maintaining efficient utilization of resources.
By splitting our application into these distinct, self-contained services, we gain the ability to independently develop, deploy, and scale each component. This approach enhances maintainability and resilience, aligning well with modern best practices for cloud-native applications.
Code snippets
Database schema design
User Schema
Payment schema
Restaurant schema
Notification Schema
similarly one can design the remaining schemas…
writing each and every details is out of scope here but to get the gist of this let’s write some key Implementations
Node.js Implementation
user service
Payment Service
Notification service
Example of Dockerfile
Python Implementation
User Service
Payment Service
Notification Service
Kubernetes Deployment
This way our cloud cluster is ready to be deployed using Kubernetes , if you are not familiar with a thing or two , you can always look for answers online and keep work going. so that’s a wrap on our microservice Journey! Navigating through these modular marvels has been like trying to debug a spaghetti code only this time, the spaghetti is neatly organized into containers.
A huge thanks to everyone who joined me on this microservice journey. May your APIs be ever responsive, your data ever consistent, and may your code be as bug-free as a well-tested deployment!