API Security: A Deep Dive into Authentication and Authorization
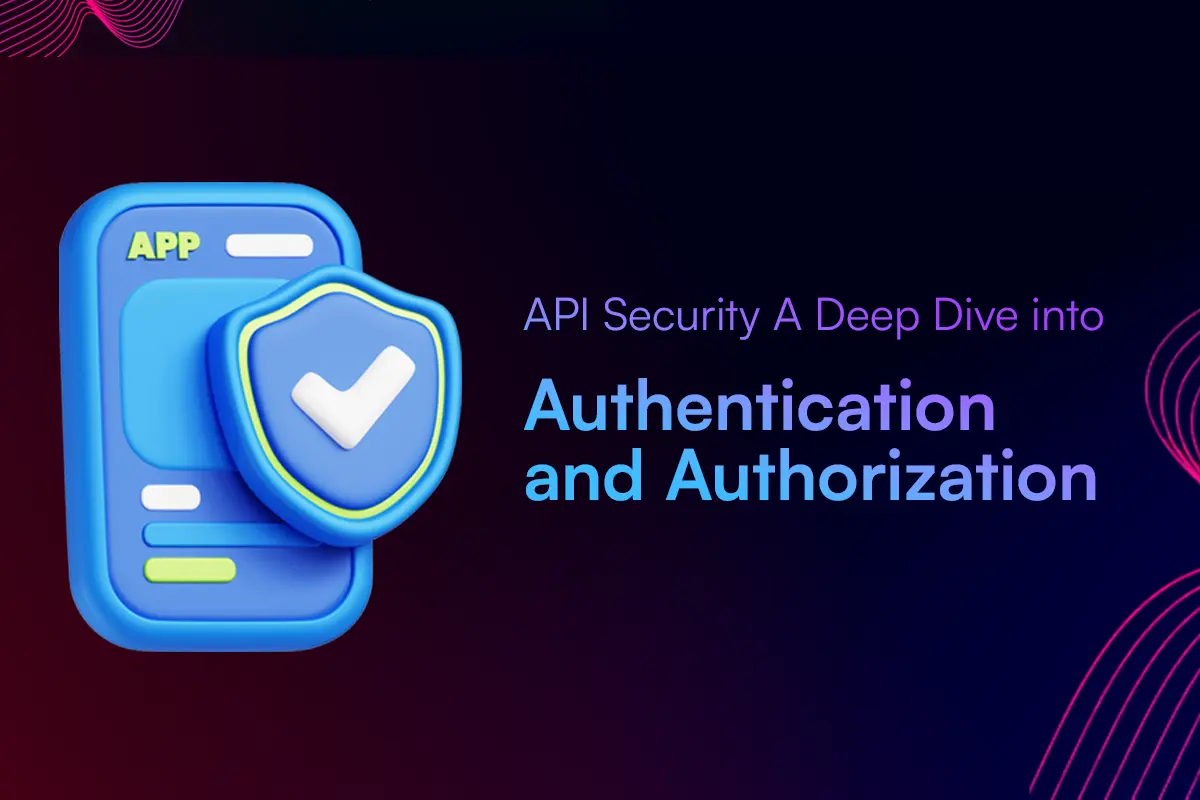
Introduction to API Security
API security is essential for Protecting data, preventing unauthorized access which can lead to business Disruption, Reputation loss, Financial losses . Also Api security can bring competitive advantage as people prefer more secure applications. One of the key features to prevent unauthorized access is using proper authentication and authorization techniques.
Authentication & Authorization
Authentication
Authentication is the process of verifying the identity of a user in the system. It answers “Who you are”?
Authorization
Authorization is the process to verify what a user is allowed to do after verifying identity.
Example: Lets understand Authentication and Authorization by example of zomato.
- Authentication
- User Enters Email id and Password to login and Press login button. Zomato verifies users and returns access tokens.
- Authorization
- Customer: Can view menus, place orders, track orders, view order history, provide ratings.
- Restaurant Owner: Can manage menu items, view orders, manage customer feedback, access analytics.
- Delivery Partner: Can view assigned orders, update order status, access navigation.
- Zomato Admin: Full access to all system components, including user management, restaurant onboarding, payment processing, analytics.
- Authentication
Authentication Techniques
JWT
Introduction
JWT Token contains information encoded and digitally signed. Generally used for authentication.
Structure
JWT contains three parts separated by dots e.g xxxx.yyyy.zzzz
Header
It contains type of token and algorithm used to sign token
Payload
It contains user information or any information that needs to be used by the server to authenticate and authorize users.
Signature
Signature is generated by encoding header and payload information by secret key or private key. While authenticating a token it will be decoded by the same secret key or public key to ensure that message is not modified.
Use Cases
- Authentication – JWT tokens are generally used for authentication. Enables stateless authentication as the server does not need to store sessions.
- Information Exchange – JWTs are a good way of securely transmitting information between parties, because as they can be signed. Additionally, as the signature is calculated using the header and the payload, you can also verify that the content hasn’t changed.
Pros
- Stateless – No need to store data on the server. It helps to improve scalability and performance.
- Decentralized – It can be verified by anyone who has a secret key.
- Secure – Token is signed with a token, it ensures data authenticity and integrity.
- Compact – Token are small in size so easy to transmit
Cons
- Revocation – You cannot revoke JWT token as they are stateless token
- Security Risk – If the Secret key is compromised. Anyone can verify the token.
Read: GraphQL vs REST
OAuth2
OAuth 2.0 is authorization framework which allows third parties applications to access resources from service without revealing password.
Roles
- Resource Owner: Entity that owns the protected resource and can grant access to their resources to client(Third party) applications.
- Client: The Application requesting access to protected resources. It can be web application, mobile application and server side application, living room devices. To access resources, the Client must hold the appropriate Access Token.
- Authorization Server: This server receives requests from the Client for Access Tokens and issues them upon successful authentication and consent by the Resource Owner. The authorization server exposes two endpoints:
- 1. Authorization endpoint: which handles the interactive authentication and consent of the user.
- 2. Token endpoint: Which is used to To obtain an access token for accessing resources.
- Resource Server: A server that has protected resources. It accepts and validates an Access Token from the Client and returns the appropriate resources to it.
Client Registration
Before a client can initiate the OAuth 2.0 flow, it must register with the Authorization Server. Client needs to provide Client Name, Client Type, Redirect URL, Scopes, Contact Information. In return Authorization Server Provides unique Client ID and Secret. These credentials are used for subsequent interactions.
Flow
- Authorization Request: The Client sends a request to the Authorization Server, providing the client ID and secret for identification, along with the requested scopes and a redirect URI to receive the Access Token or Authorization Code.
- Authentication and Scope Verification: The Authorization Server authenticates the Client and verifies that the requested scopes are allowed.
- Resource Owner Consent: The Resource Owner interacts with the Authorization Server to grant the requested access to the Client.
- Authorization Response: The Authorization Server redirects back to the Client’s specified redirect URI with either an Authorization Code or an Access Token, depending on the grant type. A Refresh Token may also be included.
- Resource Access Request: The Client uses the Access Token to request access to the resource from the Resource Server
Flow Diagram
OAuth2 Scopes
Scopes in oauth2 limit user or application’s access to protected resources. Applications can request scopes (it can be one or many), this information is visible in consent screen and access token issued for application will limit to scope granted.
- Example
- A fitness app requests ‘read_profile’ and ‘read_activity’ scopes to access user’s basic info and workout data from a wearable device.
Use Cases
- Third-Party Application Integration – User authorizes app to access their Google Drive data. App requests access token from Google OAuth server. App uses a token to upload files to user’s Google Drive.
- Api Authorization – Web app requests access to the user’s calendar API. User grants permission. App obtains access token and uses it to schedule meetings on user’s calendar.
- Internal Communication between microservices – Payment microservice requires access to user data from user profile microservice. Payment service obtains an access token using client credentials grant. Payment service uses a token to securely fetch required user data for processing payment.
- Single Sign On – User logs in to company intranet with corporate credentials. Google Workspace uses SAML to authenticate users without requiring separate login. Users access Gmail, Drive, and other G Suite apps seamlessly.
Note: OAuth 2.0 is NOT a replacement for user authentication. Oauth 2.0 will generate a token after authentication and authorize new requests.
Pros
- Enhance Security – Provides a robust framework for authorization, protecting user data and preventing unauthorized access
- User Experience – Single sign-on simplifies life by allowing users to access multiple online services with just one login. It’s like having a master key for your digital world, eliminating the hassle of remembering countless passwords.
- Decoupling: Separates authentication from authorization, allowing for more flexible system design.
- Standardization: Widely adopted and supported by major platforms and frameworks
- User Control: Grants users control over which applications can access their data.
Cons
- Complexity: Implementing OAuth 2.0 can be complex, especially for custom implementations.
- Token Management: Managing token lifecycles, revocation, and storage can be challenging.
- Performance Overhead: The process of exchanging authorization codes for tokens and validating tokens can introduce latency. OAuth 2.0 flows often require multiple HTTP requests, increasing the number of interactions between clients and servers
API Key
API keys are long, generated strings that used to authenticate requests to your API. They are essentially unique identifiers assigned to an application or user, enabling them to access your API
How API Key Authentication Works
- Key Generation: Unique API keys are created and assigned to applications or users.
- API Call: Clients include the API key in the request header or as a query parameter.
- Key Validation: The API server verifies the key against a database of authorized keys.
- Access Granted: If valid, the API proceeds with the request; otherwise, it’s denied.
Use Cases
- Public-facing APIs with less sensitive data.
- Internal APIs with limited security requirements.
- Applications where user identity is not critical.
Pros
- Simplicity: Easy to implement and understand.
- Efficiency: Quick authentication process.
- Suitable for Public APIs: Can be used for public APIs with less stringent security requirements.
- Rate Limiting – They can be used to implement rate limiting, preventing excessive API calls and protecting the API.
Cons
- Security Risks: API keys are like passwords and can be easily compromised if not handled carefully.
- Lack of User Identity: API keys don’t provide information about the user making the request.
- Limited Control: Difficult to revoke specific permissions or track API usage at a granular level.
- Potential for Abuse: Compromised keys can lead to unauthorized access and misuse.
Authorization Techniques
Role Based Access Control (RBAC)
Role-Based Access Control (RBAC) assigns permissions to users based on their roles within an organization ensures that only authorized individuals can perform specific actions.
How RBAC Works
- Define Roles: Create roles that represent different responsibilities within the organization. For example, Admin, Manager, Employee
- Assign Permissions: Determine the specific actions allowed for each role. An admin might have full access, while an employee might only be able to view data.
- Assign Users to Roles: Assign users to appropriate roles based on their position and responsibilities in the organization.
- Access Control: When a user attempts to perform an action, the system checks their role and associated permissions to determine if access is granted.
Pros
- Simplified Management: Centralized management of user permissions through roles.
- Enhanced Security: Reduces the risk of unauthorized access by limiting permissions based on roles.
- Flexibility: Easily adjust permissions by modifying role assignments.
- Scalability: Handles growing user and permission sets efficiently.
Cons
- Potential for Over-Permission: If not carefully implemented, users might be assigned roles with excessive permissions.
Attribute-Based Access Control (ABAC)
ABAC is like a smart gatekeeper that decides who can do what, based on different characteristics. Instead of just looking at someone’s job title (like in traditional systems), ABAC looks at many things like who the person is, what they want to do, what time it is, and where they are. This makes it possible to create very specific rules about who can access what information or do certain things.
ABAC considers multiple attributes to determine access. ABAC is a way to manage access to resources based on various attributes (characteristics) of users, resources, and the environment.
- Subject: The user or entity requesting access (e.g., user ID, department, clearance level).
- Resource: The data or system being accessed (e.g., data sensitivity, ownership, location).
- Environment: The context of the access request (e.g., time of day, location, device).
Example
An ABAC system allows a school teacher to access confidential student data from a school server only between 7 AM to 5 PM on weekdays while being within the school network, based on their role, time, location, and data sensitivity attributes
How ABAC Works
- Attribute Collection: Gather relevant attributes about the subject, resource, and environment.
- Policy Evaluation: Evaluate the collected attributes against predefined access control policies.
- Access Decision: Grant or deny access based on the policy evaluation outcome.
Pros
- Flexibility: Adapt to changing access requirements without modifying roles.
- Granularity: Precise control over access based on multiple attributes.
- Context Awareness: Make access decisions based on real-time conditions.
- Scalability: Handles complex access control scenarios effectively.
Cons
- Complexity: Implementing and managing ABAC policies can be complex.
- Performance Overhead: Evaluating multiple attributes can impact system performance.
- Policy Management: Defining and maintaining comprehensive access control policies requires careful consideration
Best Practices
Authentication
- Choose the right method:
- API Keys: Simple for basic identification, but offer limited security.
- OAuth 2.0: Ideal for user authorization and delegation.
- Token-based authentication: Offers flexibility and security, commonly used with JWT.
- Use bcrypt for password storage.
- Use multi-factor authentication(MFA) to Add an extra layer of security for critical APIs.
- Use Rate limiting to Protect against brute-force attacks and abuse.
Authorization
- Assign permissions based on user roles.
- Use attribute-based access control (ABAC) For dynamic and context-aware authorization.
- Grant only necessary permissions.
- Regularly review and update permissions to Ensure security and compliance.
Conclusion
Securing your APIs is not a one-time task but an ongoing process. By implementing strong authentication and authorization techniques, following best practices, and staying informed about emerging threats and technologies, you can significantly enhance the security of your APIs. Remember, in the world of API development, security should never be an afterthought – it should be woven into the fabric of your design from the very beginning.