Building Modern Web Applications with Node.js and React: A Step-by-Step Guide
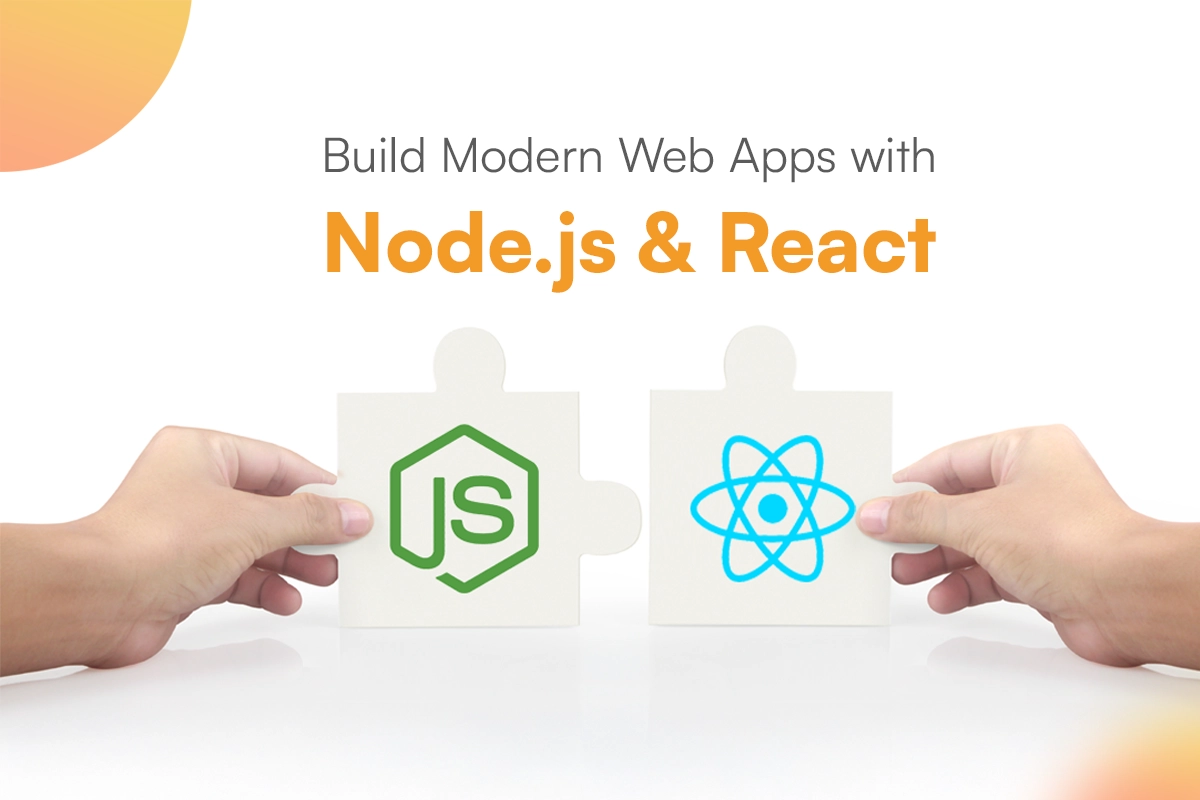
Introduction
Modern web applications need to be fast, scalable, and user-friendly. By combining the power of Node.js for the backend and React for the frontend, developers can create robust, dynamic applications that meet these demands. This guide walks you through building a modern web application using Node.js and React.
Step 1: Setting Up Your Environment
Before diving into the code, ensure your development environment is ready. Setting up the environment properly ensures smooth development and minimizes configuration-related errors later on.
Prerequisites:
- Node.js: Download and install Node.js from nodejs.org. It provides the runtime for the backend.
- Pnpm, npm or Yarn: Comes bundled with Node.js. Used for managing dependencies.
- MongoDB: Download and install MongoDB from mongodb.com. MongoDB is required to store and manage the application data.
- Code Editor: Install VS Code or your preferred editor for writing and managing your code effectively.
Create Your Project Directory:
Organize your files by creating a dedicated directory for the project:
This structure keeps your project isolated and organized.
Step 2: Setting Up the Backend with Node.js
The backend serves as the foundation for handling data and business logic. Node.js, combined with Express, makes it easier to set up a lightweight and efficient backend.
1. Initialize a Node.js Project:
This creates a package.json file to manage dependencies and scripts for your backend project.
2. Install Required Packages:
- Express: A minimal and flexible web framework for Node.js.
- CORS: Middleware for enabling Cross-Origin Resource Sharing.
- Body-parser: Parses incoming request bodies for easier handling.
- Mongoose: A library for MongoDB object modeling.
- dotenv: Allows environment variable management using a .env file.
3. Modularize Your Backend Code:
A modular structure improves maintainability and scalability by separating concerns.
Folder Structure:
This organization ensures each part of the backend is manageable and reusable.
4. Configure Environment Variables:
Create a .env file in the backend directory to manage sensitive information such as the port number:
5. Set Up the Database Connection:
Create config/db.js to manage MongoDB connection:
This script ensures a reliable connection to the MongoDB database, handling errors gracefully.
6. Define the Mongoose Model:
Models define the structure of your database documents. Create models/Item.js:
The schema specifies the structure and constraints for your data.
7. Create Routes:
Define API endpoints in routes/item-routes.js:
Routes act as entry points for client requests, linking them to the appropriate controller functions.
8. Implement Controllers:
Controllers contain the logic for handling requests. Create controllers/item-controller.js:
This modularizes request-handling logic, keeping the codebase clean.
9. Update the Main Server File:
Combine all configurations and routes in server.js:
This file acts as the entry point for the backend application, tying everything together.
10. Create a Database in MongoDB:
After installing MongoDB, start the MongoDB server using:
Then, open a new terminal and access the MongoDB shell using:
Create a new database named item-list:
You are now ready to interact with this database.
Step 3: Setting Up the Frontend with React
React enables developers to build dynamic and responsive user interfaces. Setting up a structured frontend ensures scalability.
1. Create a React App:
Initialize a new React project:
This scaffolds a React project with a pre-configured structure and tools.
2. Install Required Packages:
- react-router-dom: react-router-dom is a routing library for React that enables dynamic routing and navigation within single-page applications
- Web-vitals: web-vitals is a library by Google that measures key performance metrics like LCP, FID, and CLS to help developers enhance user experience in web applications.
3. Organize React Project Structure:
Structure your frontend for maintainability:
Folder Structure:
Organizing files by functionality improves clarity and simplifies development.
4. Configure Environment Variables:
Create a .env file in the backend directory to manage sensitive information such as the port number:
5. Create an API Service:
Centralize API interactions in src/services/api.js:
This isolates API logic, making it reusable and easier to test.
6. Create Components:
- Home Component: src/components/Home.js
- About Component: src/components/About.js
- ItemList Component: src/components/ItemList.js
7. Add Routes:
Create src/routes/AppRoutes.js to define application routes:
Update src/App.js to use routes:
Step 4: Running the Application
Once everything is set up, follow these steps to run the application:
1. Start the Backend:
Navigate to the backend directory and start the server:
This starts the Node.js backend at http://localhost:5000.
2. Start the Frontend:
Navigate to the client directory and start the React development server:
This starts the React app at http://localhost:3000.
3. Access the Application:
- Open a browser and navigate to http://localhost:3000 to view the React frontend.
- The frontend will communicate with the backend for API requests.
- Open http://localhost:3000/items to see item list page
By following these steps, you now have a fully functional modern web application powered by Node.js and React.
Conclusion
By following this guide, you have successfully built a modern web application using Node.js and React. You’ve learned how to set up a scalable backend, create a dynamic frontend, and connect them to work seamlessly. This modular and structured approach ensures your application is maintainable and ready for future enhancements. Happy coding!